How to Add ConfirmationDialog in iOS SwiftUI
Confirmation dialogs are a staple in user interface design, often used to get a user’s approval before performing a critical action. SwiftUI has a way to create these dialogs with the ConfirmationDialog
element.
In this blog post, we’ll explore how to use ConfirmationDialog
in SwiftUI, complete with code snippets and explanations.
What is a SwiftUI ConfirmationDialog?
A ConfirmationDialog
in SwiftUI is a dialog box that appears to confirm an action. It usually contains a title, a message, and one or more buttons to accept or cancel the action. It’s a straightforward way to get user confirmation for tasks like deleting files, logging out, or other irreversible actions.
Basic Usage of ConfirmationDialog
Create a State Variable
First, you’ll need a state variable to control the visibility of the dialog.
@State private var showConfirmation = false
The @State
variable showConfirmation
will determine whether the dialog is visible. When set to true, the dialog will appear.
Trigger the Dialog
Create a button that will trigger the dialog when tapped.
Button("Delete File") {
showConfirmation = true
}
This button, labeled “Delete File,” sets showConfirmation
to true when clicked, which will display the dialog.
Display the Dialog
Finally, use the confirmationDialog
modifier to display the dialog.
.confirmationDialog("Are you sure?", isPresented: $showConfirmation) {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}
The confirmationDialog
modifier takes a title (“Are you sure?”) and a binding to the showConfirmation
state variable. Inside the dialog, we define two buttons for user interaction.
Complete Code
Following is the complete code.
import SwiftUI
struct ContentView: View {
@State private var showConfirmation = false
var body: some View {
Button("Delete File") {
showConfirmation = true
}
.confirmationDialog("Are you sure?", isPresented: $showConfirmation) {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}
}
}
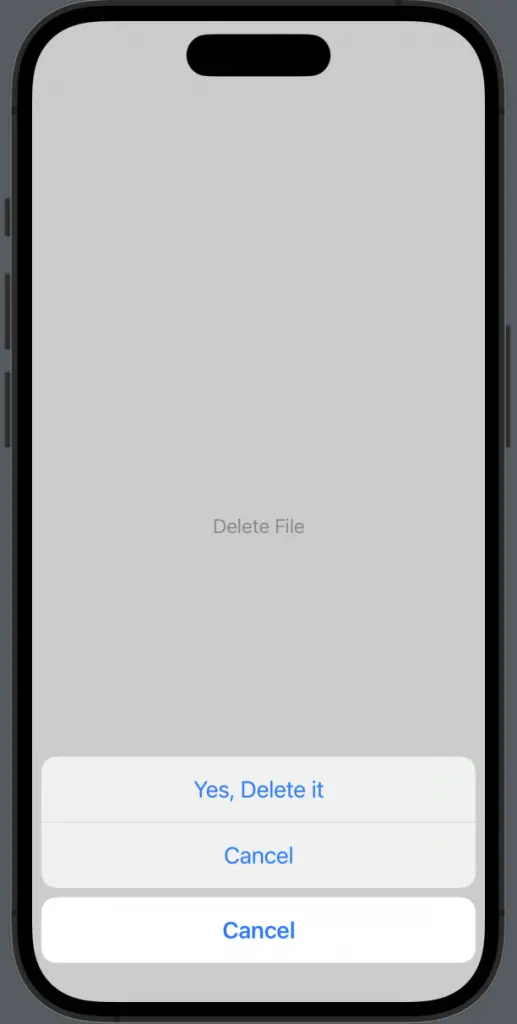
Customize ConfirmationDialog
Adding a Message
You can include a message in the dialog to provide more context.
.confirmationDialog("Are you sure?", isPresented: $showConfirmation, actions: {
Button("Yes, Delete it") {
// Perform delete action
}
Button("Cancel") {
// Cancel action
}
}) {
Text("You are about to delete a file. This action cannot be undone.")
}
The confirmationDialog
modifier now includes a trailing closure that contains a Text
element. This text serves as the message in the dialog.
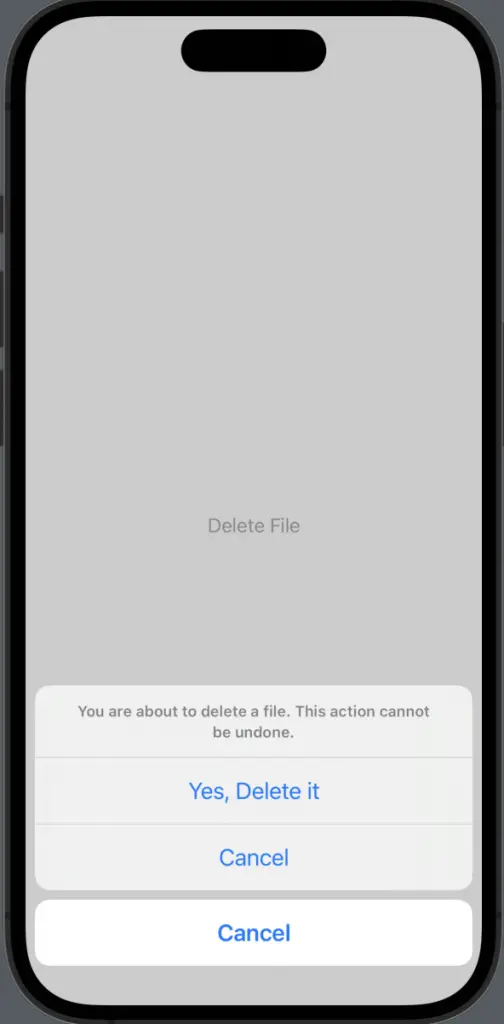
The ConfirmationDialog
in SwiftUI provides a simple yet powerful way to get user confirmation for various actions. With options for customization and advanced usage, it’s a versatile tool for enhancing user interaction in your apps.
One Comment