How to Create Gradient Background in Android Jetpack Compose
Color gradients are helpful in enhancing the beauty of your mobile app. Let’s learn how to create a background with the color gradient in Jetpack Compose.
The color gradient is part of the graphics. The Brush class comes in handy to create linear gradients, horizontal gradients, vertical gradients, radial gradients, etc.
Linear Gradient in Jetpack Compose
You can create a linear gradient using Brush and its linearGradient function. You also have options to choose multiple colors and offsets.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
val gradient = Brush.linearGradient(
0.0f to Color.Red,
500.0f to Color.Blue,
start = Offset.Zero,
end = Offset.Infinite
)
Box(modifier = Modifier.background(gradient).fillMaxSize())
}
See the output given below.
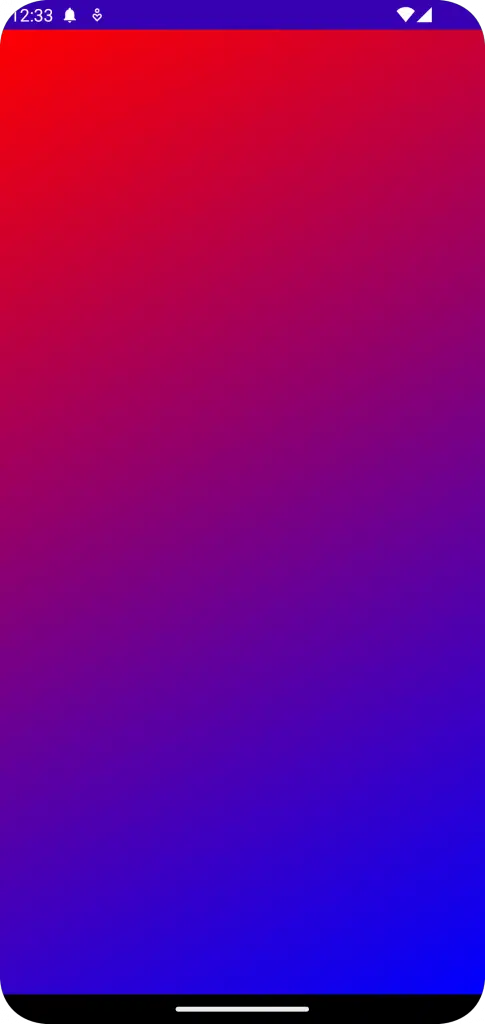
Horizontal Gradient in Jetpack Compose
You can create horizontal gradient background using Brush and the function horizontalGradient.
@Composable
fun Example() {
val gradient = Brush.horizontalGradient(
0.0f to Color.Red,
1.0f to Color.Blue,
startX = 0.0f,
endX = 1000.0f
)
Box(modifier = Modifier.background(gradient).fillMaxSize())
}
Now, you can see the horizontal gradient of red and blue colors with the given offsets.
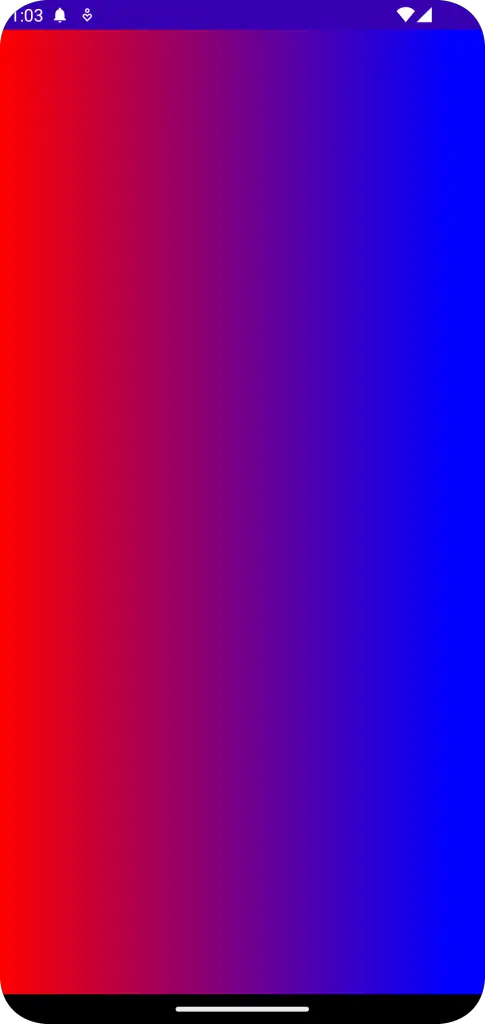
Vertical Gradient in Jetpack Compose
The Brush class and its verticalGradient functions help you to show vertical gradient background.
@Composable
fun Example() {
val gradient = Brush.verticalGradient(
0.0f to Color.Red,
1.0f to Color.Blue,
startY = 0.0f,
endY = 1500.0f
)
Box(modifier = Modifier.background(gradient).fillMaxSize())
}
The output will have a vertical gradient background with red and blue colors.
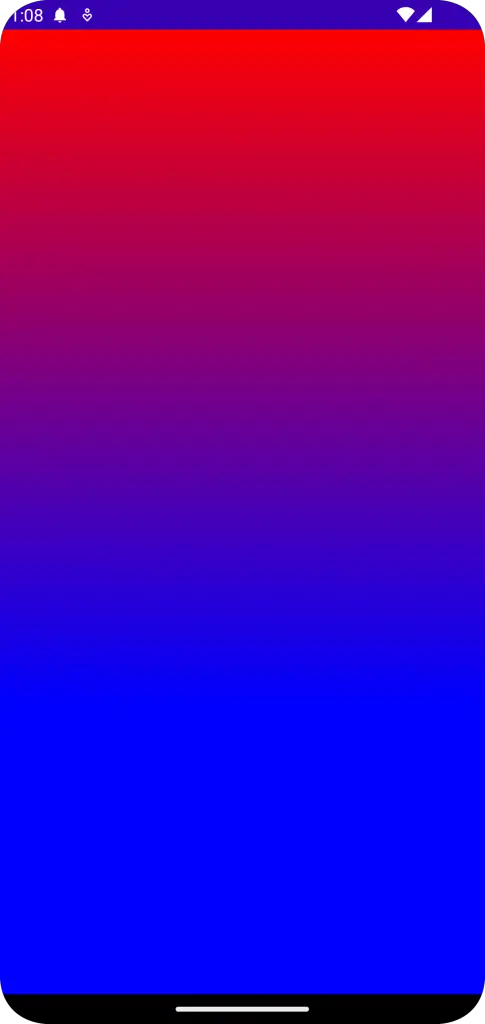
Radial Gradient in Jetpack Compose
A radial gradient is a gradient that radiates from an origin.
@Composable
fun Example() {
val gradient = Brush.radialGradient(
0.0f to Color.Red,
1.0f to Color.Blue,
radius = 1500.0f,
tileMode = TileMode.Repeated
)
Box(modifier = Modifier.background(gradient).fillMaxSize())
}
Following is the output.
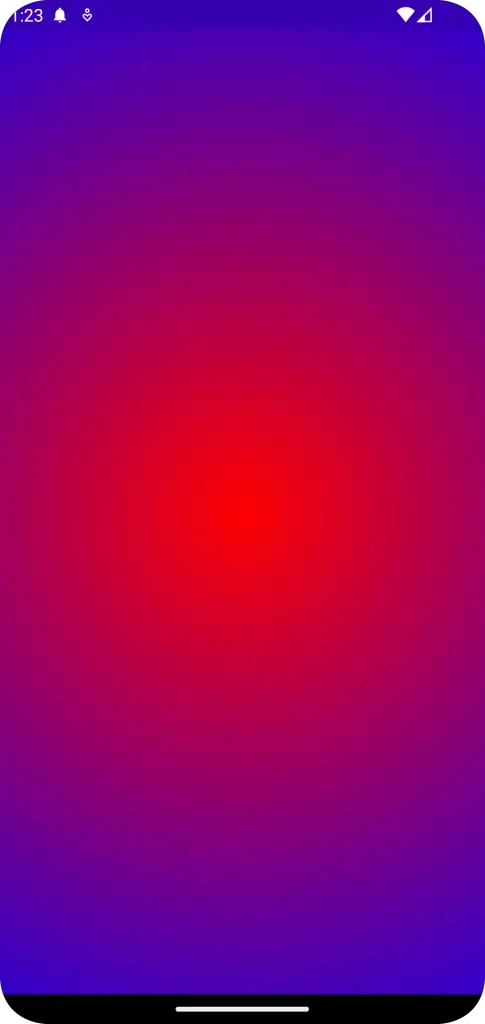
That’s how you create different gradient backgrounds in Jetpack Compose.
2 Comments