How to Control Sheet Height in iOS SwiftUI
In SwiftUI, sheets are a convenient way to present secondary views or modals. While the framework does a good job of automatically sizing these sheets based on their content, there are times when you might want to specify a custom height.
In this blog post, we’ll explore how to control the height of a sheet in SwiftUI using the .presentationDetents modifier.
Set a Fixed Height for Sheet
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.height(250)])
}
}
}
Code Explanation
.presentationDetents([.height(250)])
: This modifier allows you to set a fixed height for the sheet. In this example, the sheet will have a height of 250 points.
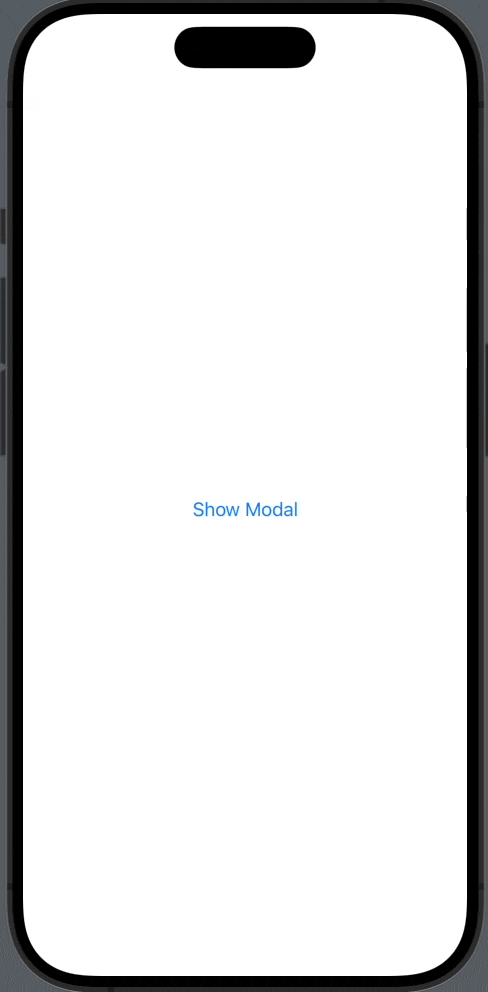
Set a Minimum and Maximum Sheet Height
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.height(250),.height(500)])
}
}
}
Code Explanation
.presentationDetents([.height(250),.height(500)])
: This modifier allows you to set both a minimum and maximum height for the sheet. The sheet will initially appear at the minimum height (250 points) and can be dragged up to the maximum height (500 points).
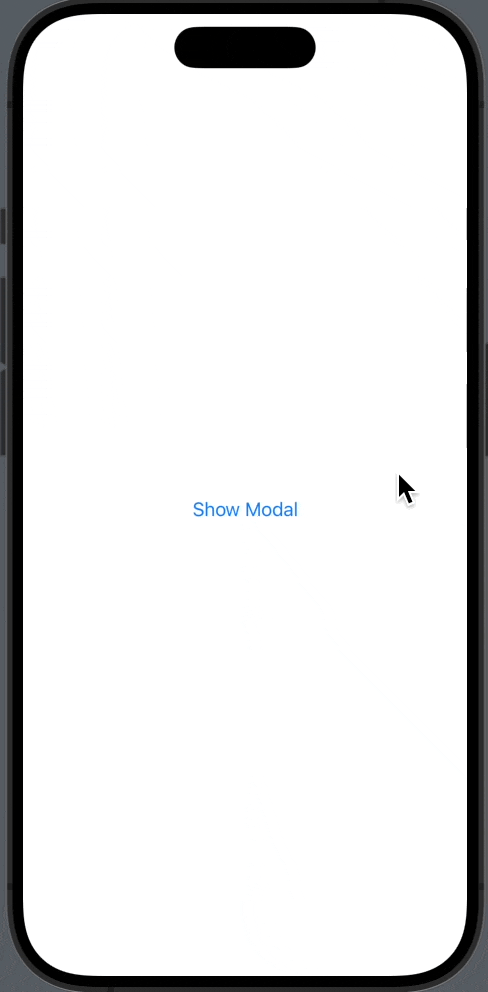
If you want to create half screen sheet then see this SwiftUI half-screen sheet tutorial.
Why Control Sheet Height?
Controlling the height of a sheet can be useful for various reasons:
- User Experience: A custom height can make the sheet more user-friendly, especially if the default height doesn’t suit your content.
- Design Consistency: If you have a design language that specifies modal sizes, setting a custom height ensures consistency across your app.
- Content Fit: Sometimes, you might have content that requires a specific amount of space to be displayed effectively. Custom heights can help in such cases.
The .presentationDetents modifier in SwiftUI provides a straightforward way to control the height of a sheet. Whether you need a fixed height or a range between a minimum and maximum, this modifier has got you covered. It’s a useful tool for making your sheets more adaptable and user-friendly.
3 Comments