How to Dismiss FullScreenCover in iOS SwiftUI
One of the most engaging ways to present information or collect input from the user in an iOS app is through modals. SwiftUI gives us the fullScreenCover
function for displaying full-screen modals. But how do you dismiss them?
In this blog post, we’ll explore different methods to dismiss full-screen modals in SwiftUI.
The Basics: fullScreenCover
Before we dive into dismissing modals, let’s recap how to create a full-screen modal using SwiftUI’s fullScreenCover
.
@State private var isModalPresented = false
var body: some View {
Button("Show Full Screen Modal") {
isModalPresented.toggle()
}
.fullScreenCover(isPresented: $isModalPresented) {
Text("This is a full-screen modal")
}
}
Here, we define a state variable isModalPresented
to control the presentation of the modal. We then use fullScreenCover
to present the modal view.
The onDismiss Parameter
SwiftUI allows you to execute code when the modal is dismissed using the onDismiss
closure parameter.
.fullScreenCover(isPresented: $isModalPresented, onDismiss: {
print("Modal dismissed")
}) {
Text("This is a full-screen modal")
}
This is useful for any clean-up activities or post-dismissal operations.
Manual Dismissal: Using a Button
You can add a button inside your modal view that changes the isModalPresented
state variable to false
.
.fullScreenCover(isPresented: $isModalPresented, onDismiss: {
print("Modal dismissed")
}) {
VStack {
Text("This is a full-screen modal")
Button("Dismiss") {
isModalPresented = false
}
}
}
By setting isModalPresented
to false
, you manually dismiss the modal.
Complete Code
Following is the complete example.
import SwiftUI
struct ContentView: View {
@State private var isModalPresented = false
var body: some View {
Button("Show Full Screen Modal") {
isModalPresented.toggle()
}
.fullScreenCover(isPresented: $isModalPresented, onDismiss: {
print("Modal dismissed")
}) {
VStack {
Text("This is a full-screen modal")
Button("Dismiss") {
isModalPresented = false
}
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
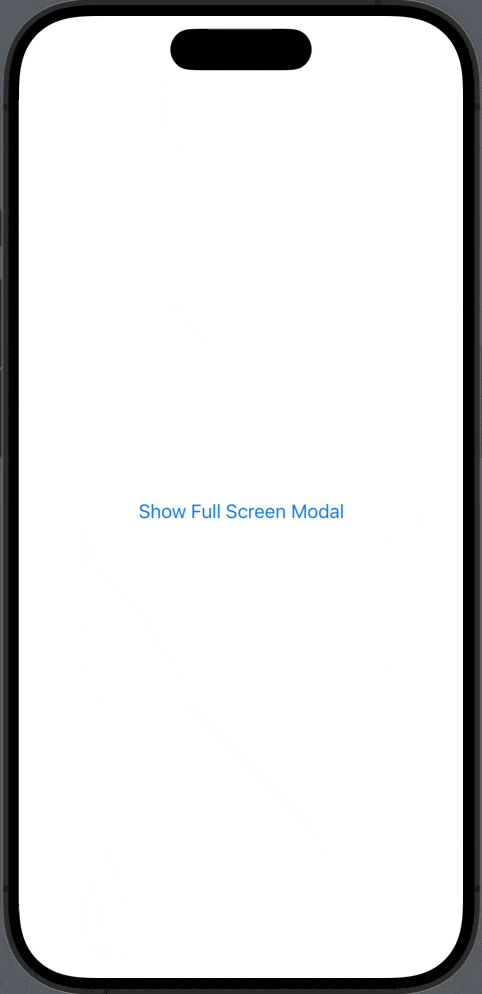
Dismissing a full-screen modal in SwiftUI is pretty straightforward. You have multiple options, ranging from using the onDismiss
parameter with a manual button. You can also choose swipe to dismiss fullScreenCover with this logic. Depending on your use case, you can opt for the method that fits your needs the best.
One Comment