How to Add List Inside a Sheet Properly in SwiftUI
In SwiftUI, sheets are a convenient way to present secondary views or modals. While adding a list inside a sheet is straightforward, it can introduce some interaction issues, especially when the sheet is resizable.
In this blog post, we’ll explore how to add a list inside a sheet properly in SwiftUI, ensuring smooth scrolling and interaction.
The Problem: Scrolling vs Dragging
When you add a list inside a resizable sheet, you may encounter an issue where the sheet gets dragged instead of scrolling the list when the sheet is at medium size.
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
List(1...20, id: \.self) { index in
Text("Item \(index)")
}.presentationDetents([.medium, .large])
}
}
}
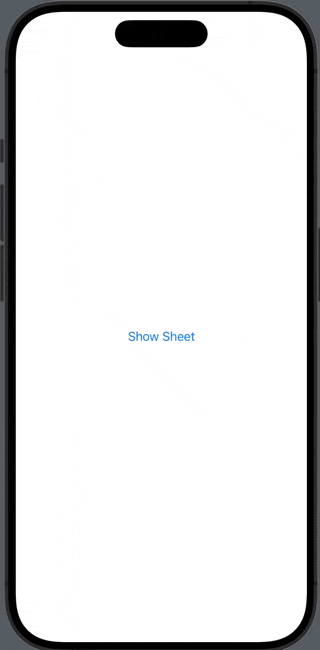
The Solution: Enable Scrolling
To solve this issue, you can use the .presentationContentInteraction
modifier and set its value to .scrolls
.
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
List(1...20, id: \.self) { index in
Text("Item \(index)")
}.presentationDetents([.medium, .large])
.presentationContentInteraction(.scrolls)
}
}
}
Code Explanation
.presentationContentInteraction(.scrolls)
: This modifier allows the list to scroll properly when the sheet is at medium size, instead of dragging the sheet itself.
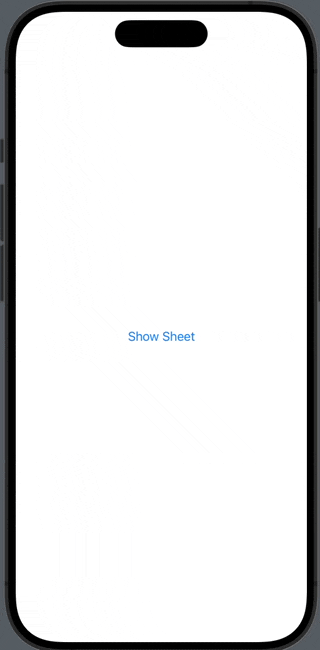
Why Use .presentationContentInteraction(.scrolls)
?
Here are some reasons why you might want to use this modifier:
- User Experience: It provides a better user experience by allowing the list to scroll smoothly.
- Functionality: It ensures that the list inside the sheet functions as expected, without any interaction issues.
- Design Consistency: It maintains the design consistency of your app by making sure that all elements behave as they should.
Adding a list inside a sheet in SwiftUI is a common requirement, but it can introduce interaction issues. By using the .presentationContentInteraction(.scrolls)
modifier, you can ensure that the list scrolls properly, enhancing the user experience and maintaining design consistency.