How to Create Rectangle Shape in SwiftUI
In SwiftUI, the Rectangle shape is a fundamental building block for UI design. It’s simple yet incredibly versatile. This post is dedicated to showing you the full potential of the Rectangle shape in SwiftUI.
The Basics of SwiftUI Rectangle
Let’s start with the foundation. A Rectangle in SwiftUI is a view that draws a rectangular shape to the frame you specify.
import SwiftUI
struct ContentView: View {
var body: some View {
Rectangle()
.frame(width: 200, height: 100)
}
}
This code snippet creates a Rectangle with a width of 200 points and a height of 100 points. If you don’t specify a color, it will default to black.
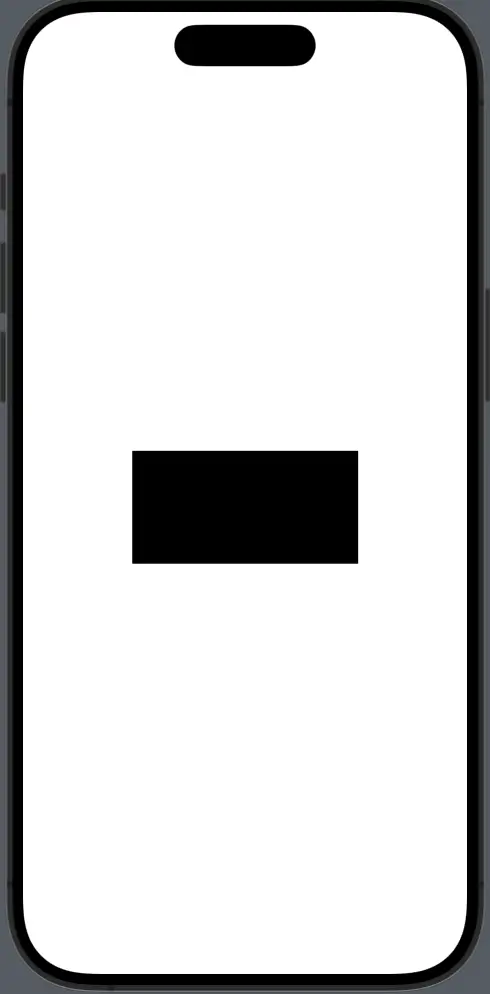
Customize with Fill Color
Color is essential for distinguishing your Rectangle. You can fill it with a solid color using the fill()
modifier.
Rectangle()
.fill(Color.blue)
.frame(width: 200, height: 100)
The Rectangle now will appear filled with blue color. It’s a straightforward way to give your shape some personality.
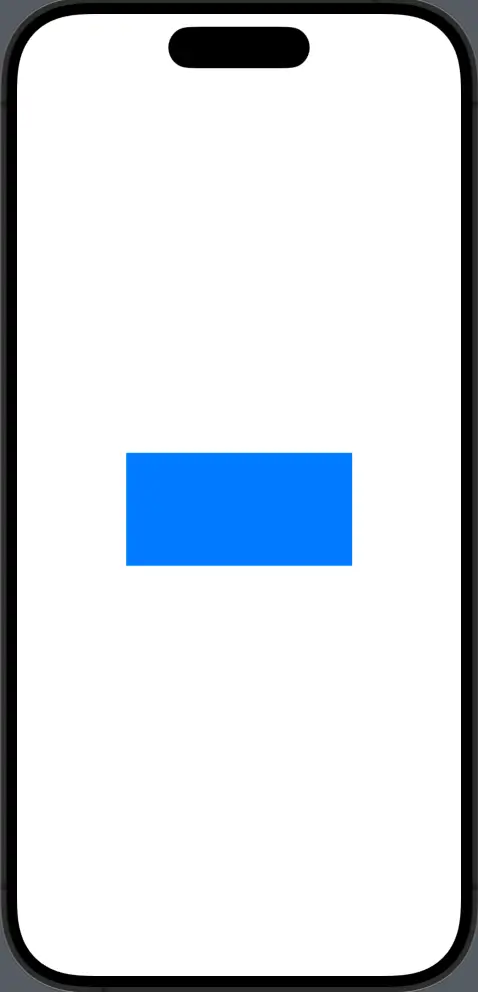
Borders and Strokes
To make the Rectangle stand out or to create an outline, you can use a stroke.
Rectangle()
.stroke(Color.red, lineWidth: 3)
.frame(width: 200, height: 100)
Here, the Rectangle has a red border with a line width of 3 points. It fills the same space, but only the border is visible.
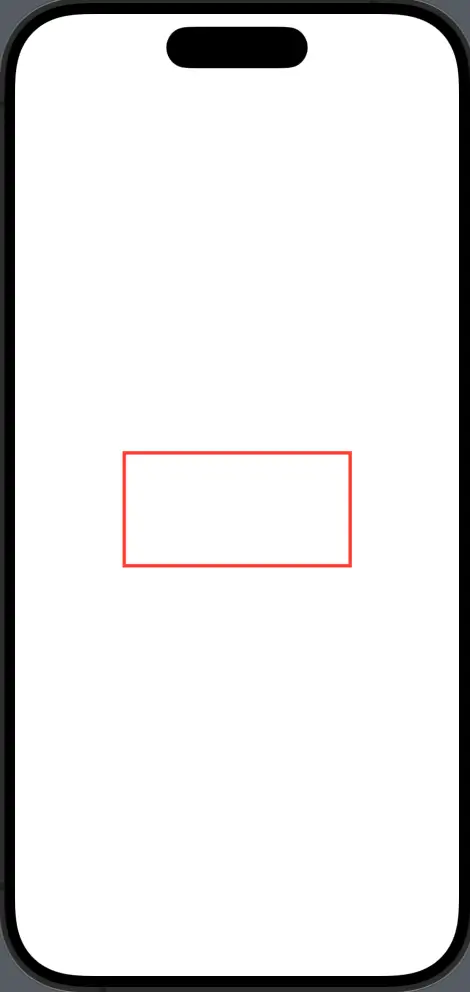
Rectangle with Rounded Corners
A Rectangle with sharp corners is not always what you need. You can round the corners with the cornerRadius()
modifier.
Rectangle()
.fill(Color.green)
.frame(width: 200, height: 100)
.cornerRadius(10)
The cornerRadius()
modifier gives the Rectangle rounded corners, softening its appearance.
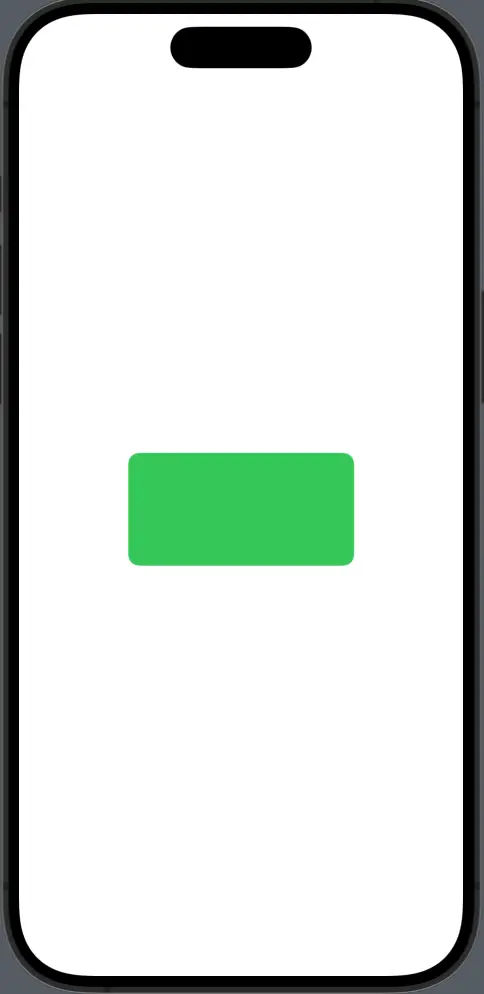
Rectangle with Shadows
Shadows can add depth to your Rectangle, making it more visually interesting.
Rectangle()
.fill(Color.purple)
.frame(width: 200, height: 100)
.shadow(radius: 5)
The shadow()
modifier applies a shadow with a radius of 5 points around the Rectangle.
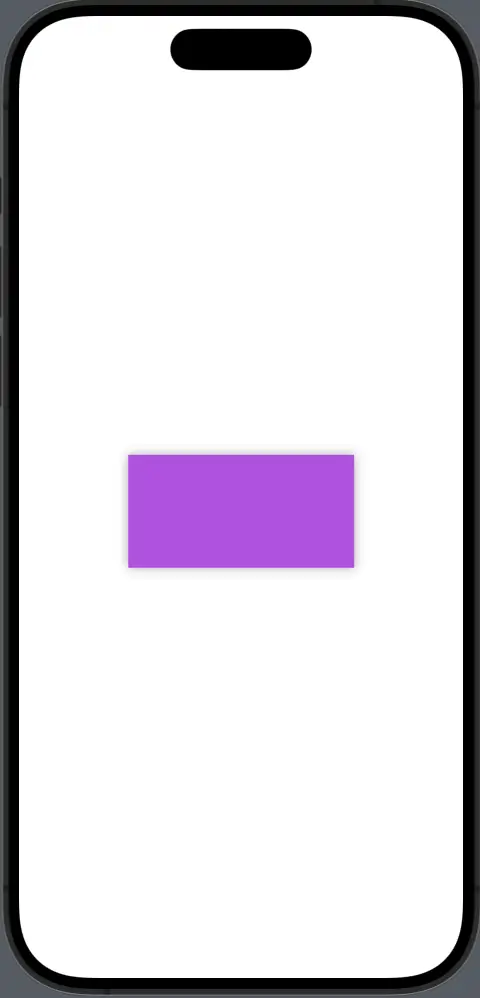
The Rectangle shape in SwiftUI is a versatile tool. By customizing its color, border, corner radius, shadow, and gradient, you can create a variety of UI elements that are both functional and visually appealing.