How to Create AnnotatedString in Android Jetpack Compose
Jetpack Compose, the modern toolkit for building native Android UI, simplifies the task of creating beautiful and interactive UIs. It’s also incredibly flexible when it comes to creating and formatting text. One powerful tool it provides for this purpose is the AnnotatedString.
An AnnotatedString is a string that has additional metadata attached to segments of its text. This metadata can be used to apply rich formatting to parts of the string, such as changing the color, font, or style of certain sections, or adding hyperlinks or custom actions. Let’s explore how to use it.
Create and Display AnnotatedString
In Jetpack Compose, an AnnotatedString is created using the buildAnnotatedString function. Here’s an example of how to create an AnnotatedString:
@Composable
fun AnnotatedStringExample() {
Column{
val text = buildAnnotatedString {
withStyle(style = SpanStyle(color = Color.Red)) {
append("Red")
}
append(" and ")
withStyle(style = SpanStyle(fontWeight = FontWeight.Bold, fontSize = 20.sp)) {
append("Bold")
}
}
Text(text = text)
}
}
In this example, we’ve created a string with two different sections, each with its own style. The first section, “Red”, is displayed in red, and the second section, “Bold”, is displayed in bold.
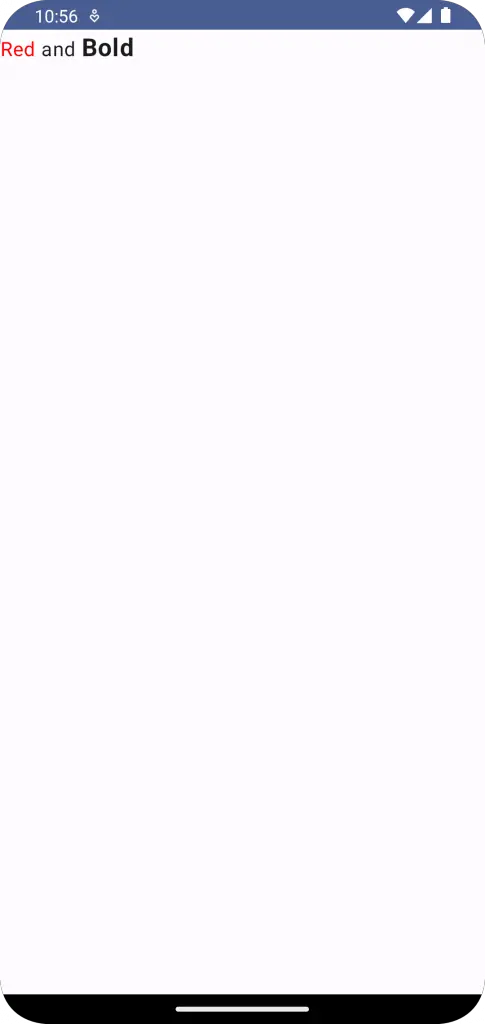
How to Add Annotations
In addition to styling text, we can also add annotations to an AnnotatedString. Annotations are pieces of metadata that can be associated with a section of text:
@Composable
fun AnnotatedStringExample() {
Column{
val text = buildAnnotatedString {
withStyle(style = SpanStyle(color = Color.Red)) {
append("Red")
}
append(" and ")
withStyle(style = SpanStyle(fontWeight = FontWeight.Bold, fontSize = 20.sp)) {
append("Bold")
}
addStringAnnotation(tag = "CustomTag", annotation = "CustomValue", start = 0, end = 3)
}
Text(text = text)
}
}
In this example, we’ve added an annotation to the first three characters of the string. This annotation has a tag of “CustomTag” and a value of “CustomValue”.
How to Use Annotations
Annotations can be used to perform custom actions. For example, we can use them to create interactive text:
@Composable
fun AnnotatedStringExample() {
Column{
val text = buildAnnotatedString {
withStyle(style = SpanStyle(color = Color.Red)) {
append("Red")
}
append(" and ")
withStyle(style = SpanStyle(fontWeight = FontWeight.Bold, fontSize = 20.sp)) {
append("Bold")
}
addStringAnnotation(tag = "CustomTag", annotation = "CustomValue", start = 0, end = 3)
}
Text(
text = text,
modifier = Modifier.clickable {
text.getStringAnnotations("CustomTag", start = 0, end = 3)
.firstOrNull()
?.let {
// Perform some action
}
}
)
}
}
In this example, when the user clicks on the Text, we retrieve the annotation for the first three characters of the string and perform an action if an annotation with the tag “CustomTag” is found.
The AnnotatedString provides a flexible way to create richly-formatted, interactive text in Jetpack Compose. With it, you can easily add style and interactivity to your app’s text.