How to Set LazyVGrid Background Color in iOS SwiftUI
SwiftUI offers an array of tools for developers to create stunning and efficient user interfaces. Among these, LazyVGrid
stands out for its simplicity in creating grid-based layouts. But what if you want to customize your LazyVGrid
with a background color?
This blog post will guide you through the process of adding a background color to your LazyVGrid
.
What is a LazyVGrid?
Before jumping into how to add background colors, let’s quickly cover what a LazyVGrid
is. The LazyVGrid
is a container that arranges its child views into a grid that grows vertically, loading items on demand as the user scrolls through the view.
struct ContentView: View {
let columns = [
GridItem(.flexible()),
GridItem(.flexible()),
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 20) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(height: 100)
.cornerRadius(10)
}
}
.padding()
}
}
}
This basic LazyVGrid
generates a vertical grid filled with colored rectangles.
Add Background Color to LazyVGrid
To set a background color for the LazyVGrid
, you can simply use the .background()
modifier and pass in a color. The Color
struct provides a palette of colors to choose from.
struct ContentView: View {
let columns = [
GridItem(.flexible()),
GridItem(.flexible()),
]
let colors: [Color] = [.red, .green, .blue, .orange, .yellow, .pink, .purple, .gray]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 20) {
ForEach(colors, id: \.self) { color in
Rectangle()
.fill(color)
.frame(height: 100)
.cornerRadius(10)
}
}
.padding()
.background(Color.black)
}
}
}
In this code snippet, the LazyVGrid
has a black background color. The result is a grid of colorful rectangles on a solid black backdrop.
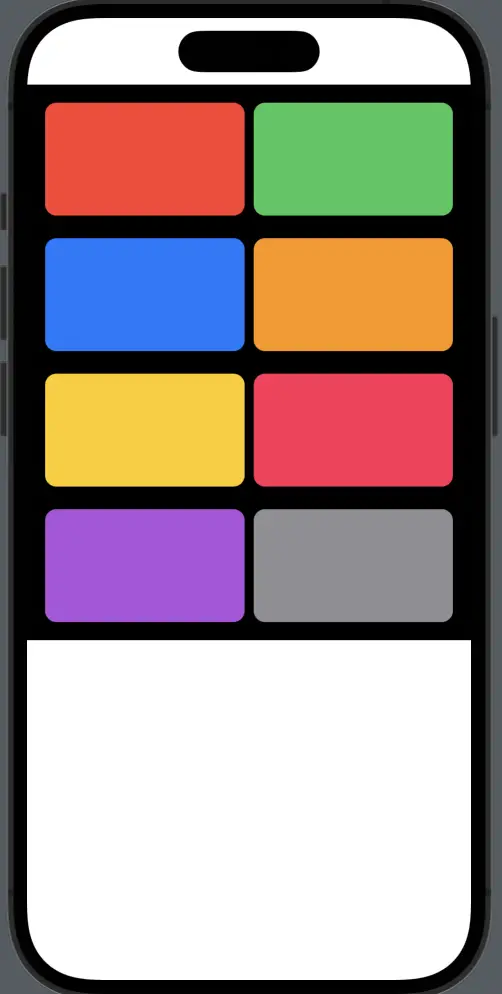
Adding a background color to LazyVGrid
in SwiftUI is straightforward with the use of the .background()
modifier. This is just one of the many ways to customize LazyVGrid
to make your app look more appealing and fit your design needs.