How to Create Bottom Sheet in iOS SwiftUI
Bottom sheets are a popular UI element in modern mobile applications. They are used to display additional information or actions that are related to the current screen.
In SwiftUI, creating a bottom sheet is quite straightforward, thanks to the .sheet
and .presentationDetents
modifiers. In this blog post, we’ll explore different ways to create a bottom sheet in SwiftUI.
Create a Draggable Bottom Sheet
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Bottom Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm the bottom sheet!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium, .large])
}
}
}
Code Explanation
.presentationDetents([.medium, .large])
: This modifier specifies that the sheet will have two detents, medium and large. This allows the sheet to be draggable from half-screen to full-screen.
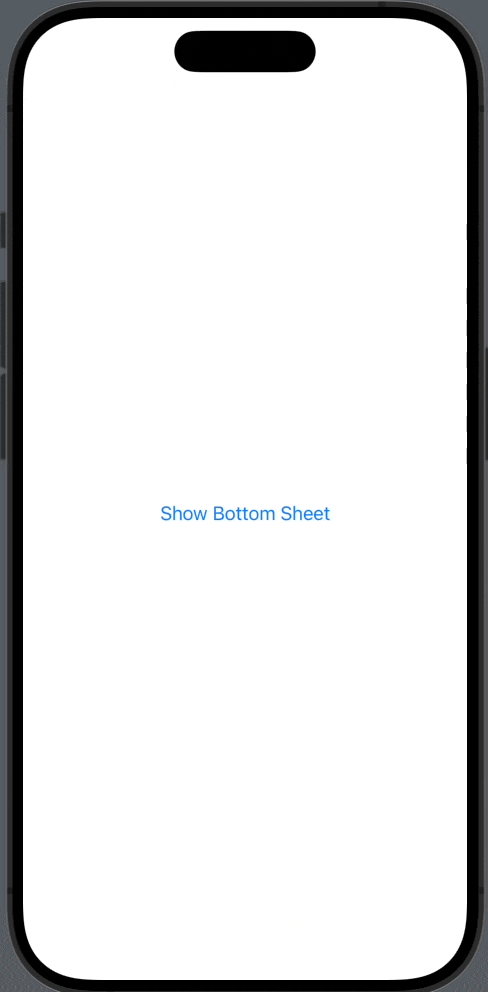
Create Bottom Sheet with Fixed Height
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Bottom Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm the bottom sheet!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.height(250)])
}
}
}
Code Explanation
.presentationDetents([.height(250)])
: This modifier sets a fixed height of 250 points for the bottom sheet.
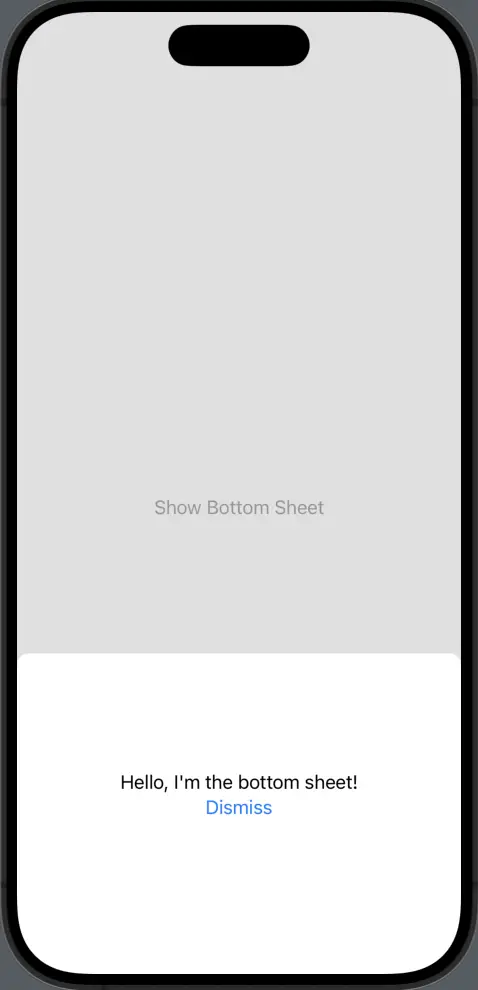
Create Bottom Sheet with Fractional Height
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Bottom Sheet") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm the bottom sheet!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.fraction(0.2)])
}
}
}
Code Explanation
.presentationDetents([.fraction(0.2)])
: This modifier sets the height of the bottom sheet to be 20% of the available height.
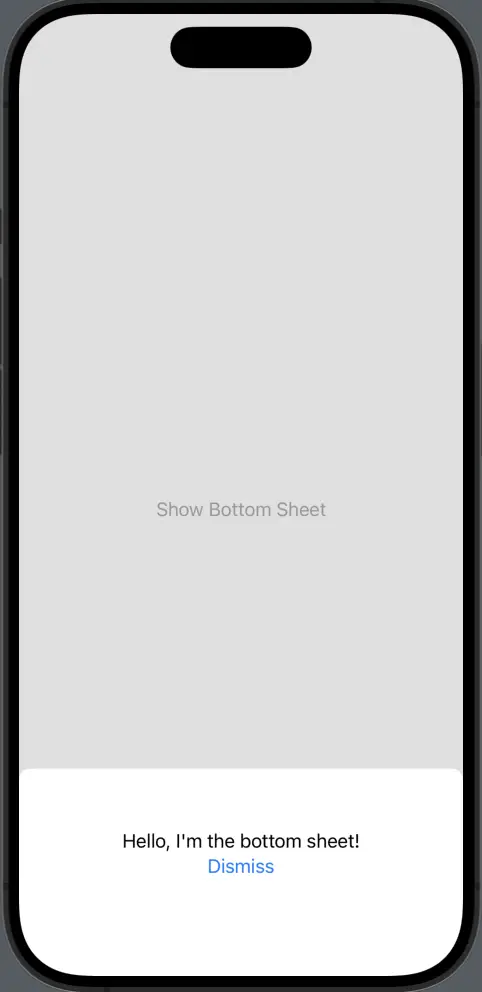
Why Use a Bottom Sheet?
- User Experience: Bottom sheets are less intrusive and allow users to maintain context with the underlying content.
- Flexibility: They are highly customizable, allowing you to set fixed, fractional, or multiple heights.
- Design Consistency: Using bottom sheets can help maintain a consistent design language across your app.
Creating a bottom sheet in SwiftUI is a straightforward process, thanks to the .sheet
and .presentationDetents
modifiers. Whether you want a draggable sheet, a sheet with a fixed height, or a sheet with a fractional height, SwiftUI has got you covered.