How to Manage TextField Focus in iOS SwiftUI
In app development, handling the focus of text input controls is critical. Focus management helps to enhance the user experience by guiding the user through forms or improving accessibility.
In SwiftUI, managing the focus on a TextField
is made simpler with the @FocusState
property wrapper. This blog post explores how to control and observe the focus state in SwiftUI.
@FocusState Property Wrapper
The @FocusState
property wrapper is used to manage the focus state of a control. You can declare a variable with this property wrapper and bind it to a TextField
, thereby gaining control over its focus state.
Set Focus Programmatically
Example 1: Simple Focus Management
Here’s a basic example where you can toggle the focus of a TextField
using a button.
struct ContentView: View {
@FocusState private var isFocused: Bool
var body: some View {
VStack {
TextField("Enter text", text: .constant(""))
.focused($isFocused)
Button("Toggle Focus") {
isFocused.toggle()
}
}
}
}
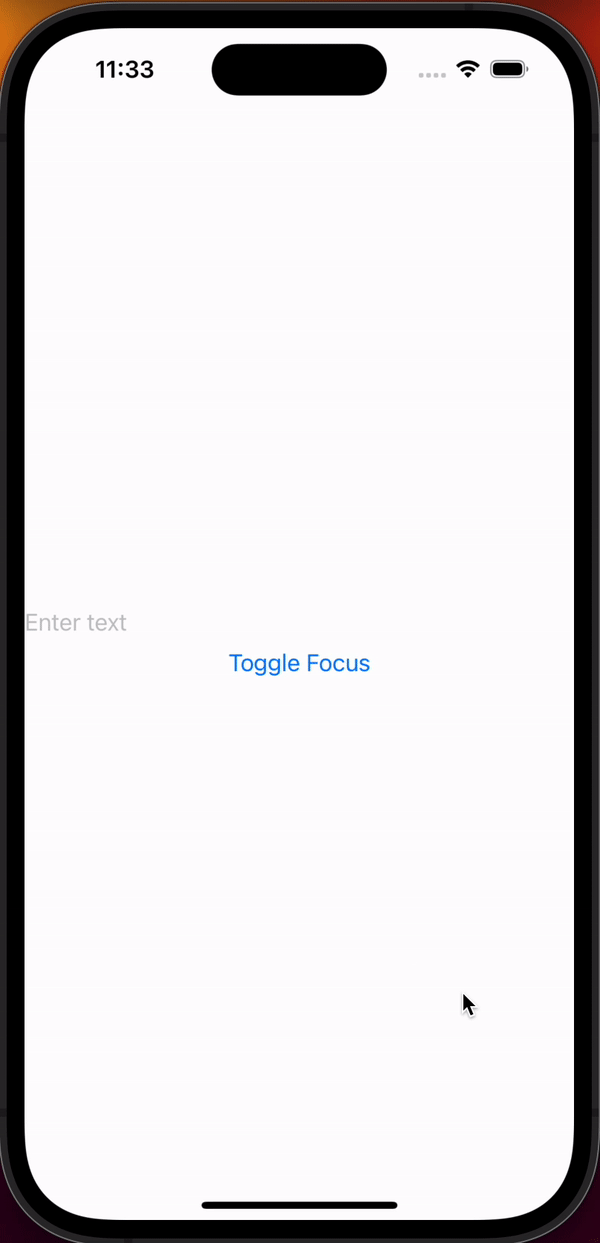
How to Handle Focus Changes
To handle changes to the focus state, you can use the onChange
modifier to observe and respond to changes.
Example 2: Observe Focus Changes
struct ContentView: View {
@FocusState private var isFocused: Bool
var body: some View {
TextField("Enter text", text: .constant(""))
.focused($isFocused)
.onChange(of: isFocused) { newValue in
print("Focus state changed to \(newValue)")
}
}
}
The @FocusState
property wrapper in SwiftUI opens doors to efficient focus management in your app. From simple focus toggling to navigation through multiple fields, and even observing changes, you have extensive control and ease of use.