How to Customize Keyboard for Email Input in iOS SwiftUI TextField
When creating a form for users to enter their email address, it’s essential to provide a user-friendly keyboard specifically tailored for email input. This ensures an easier and more accurate input process.
SwiftUI offers a straightforward way to customize the keyboard for email input. In this blog post, we will explore how to set up a TextField in SwiftUI for email input by using the email keyboard.
Set Up an Email Keyboard in SwiftUI
Create a Basic TextField for Email
Here’s a straightforward example of a TextField used for email input:
struct ContentView: View {
@State private var email = ""
var body: some View {
TextField("Enter your email", text: $email)
.keyboardType(.emailAddress)
.padding()
.autocapitalization(.none)
}
}
The code snippet above includes some vital components to ensure a smooth email input experience:
- TextField: The basic TextField element where users can enter their email. It’s bound to the
email
state variable to store the input. - keyboardType Modifier: By setting the
.emailAddress
keyboard type, SwiftUI presents the user with a keyboard optimized for entering email addresses. It includes essential characters like ‘@’ and ‘.’. - padding Modifier: This adds some space around the TextField to make it visually appealing.
- autocapitalization Modifier: Since email addresses are typically lowercase, the
.none
option is used to prevent automatic capitalization of the entered text.
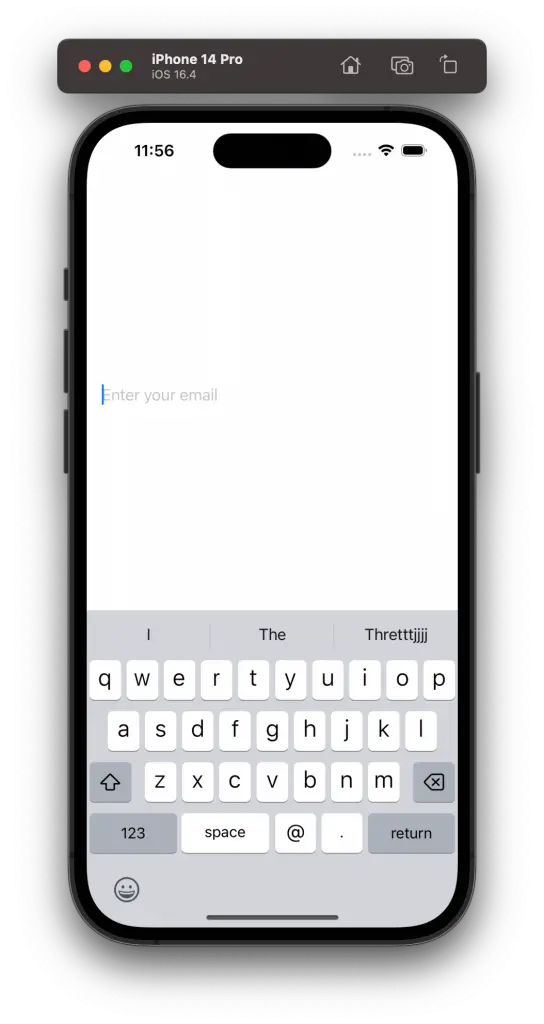
Additional Customizations
Placeholder Customization
You might want to customize the placeholder text to guide the user effectively. You can do this by providing a clear string inside the TextField initializer, like "Enter your email"
.
Adding a “Submit” Button
You could also include a “Submit” button to handle the email submission:
Button("Submit") {
print("Email submitted: \(email)")
}
.disabled(email.isEmpty || !email.contains("@"))
This code includes a basic validation that enables the button only if the email field is not empty and contains the ‘@’ character.
SwiftUI provides a seamless way to customize the keyboard for email input. By utilizing the .emailAddress
keyboard type along with other modifiers like autocapitalization
, developers can create an intuitive and user-friendly email input field.
These customizations are essential for enhancing the overall user experience, especially in forms requiring email input.