How to Create Square Shape in SwiftUI
SwiftUI provides an intuitive and powerful framework for designing UI components, and shapes are a fundamental aspect of this. Among these, the square is a basic yet versatile shape used in various design contexts, from buttons and icons to placeholders and tiles.
Let’s look into how to create and manipulate square shapes within SwiftUI.
Create a Basic Square
The simplest way to start with squares in SwiftUI is by using the Rectangle
shape and specifying equal width and height.
import SwiftUI
struct ContentView: View {
var body: some View {
Rectangle()
.frame(width: 100, height: 100)
}
}
This code snippet will produce a perfect square with a 100-point side length. The frame
modifier is crucial here; it dictates the dimensions of the shape.
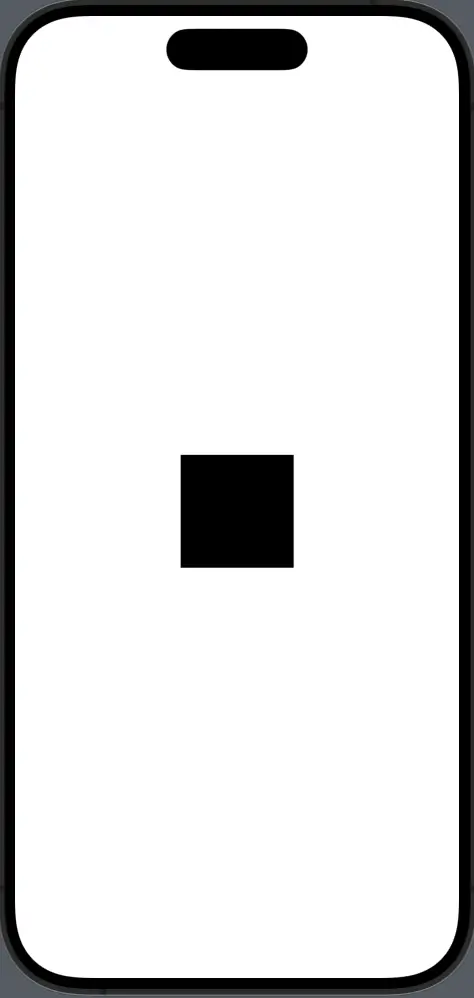
Style the Square
SwiftUI allows you to style shapes extensively with fills, borders, shadows, and more.
Rectangle()
.fill(Color.blue)
.frame(width: 150, height: 150)
.border(Color.red, width: 3)
.shadow(radius: 10)
In the above example, the square has been given a blue color, a red border, and a shadow for depth. The fill
modifier changes the interior color, while border
and shadow
add a border and shadow, respectively.
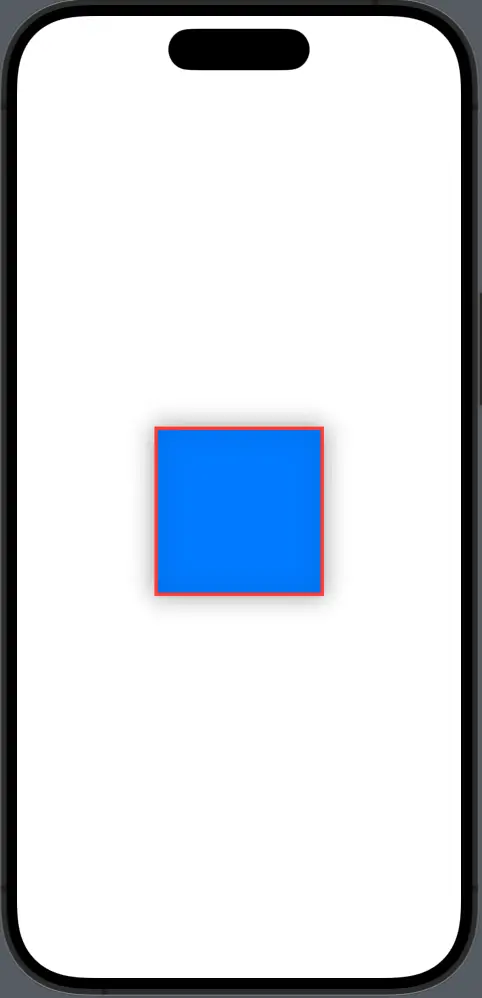
Rounded Corners
A square doesn’t have to have sharp corners. With SwiftUI, you can easily round the corners of a square.
Rectangle()
.fill(.red)
.cornerRadius(20)
.frame(width: 200, height: 200)
The cornerRadius
modifier softens the corners, transforming the square into a rounded square.
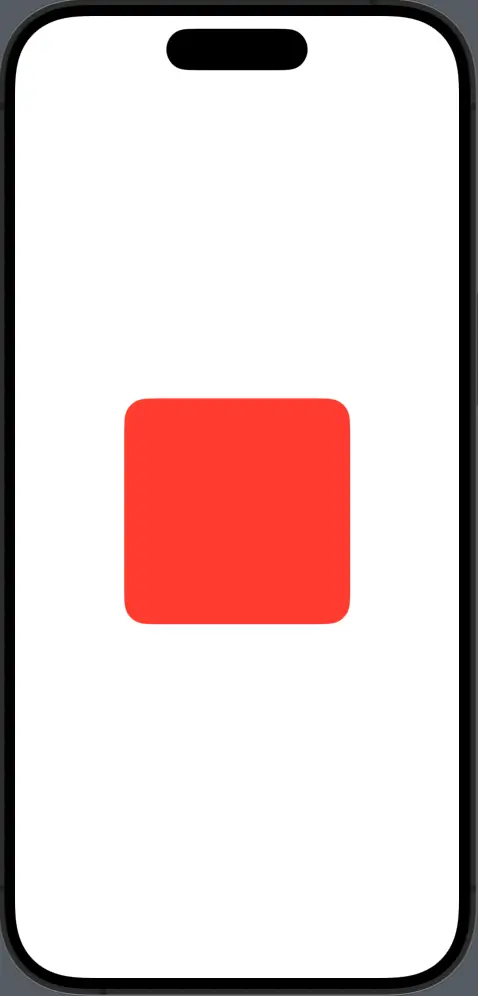
Squares with Images
You can also use squares as a clipping mask for images, creating square-shaped images.
Image("dog")
.resizable()
.aspectRatio(contentMode: .fill)
.frame(width: 150, height: 150)
.clipShape(Rectangle())
The clipShape
modifier clips the image to the shape of the rectangle, resulting in a square image.
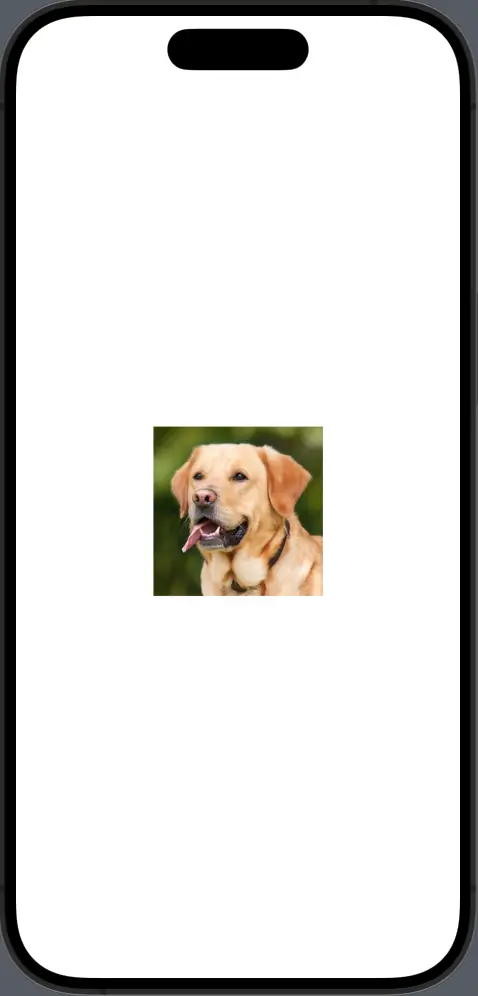
The square is a staple in design and SwiftUI makes it extremely easy to integrate into your app’s interface. Whether you’re creating a simple button, designing a grid layout, or using squares as clipping masks, SwiftUI’s tools are both simple and robust enough to achieve your design goals.
Meta Description: