How to change Color of Image in Flutter
The images come with predefined colors. Sometimes, we may need to tweak the color of images. In this Flutter tutorial, let’s discuss how to change the color of an image.
To change the color of an image, I prefer the ColorFiltered widget of Flutter. It has the colorFilter property which changes the color filter of the child. In this Flutter example, we are changing the color of the following image.

As you see, the color of the above image is red. We change the color of the above image to red using the ColorFiltered widget. See the code snippet below.
ColorFiltered(
colorFilter: const ColorFilter.mode(Colors.red, BlendMode.color),
child: Image.network(
"https://cdn.pixabay.com/photo/2019/12/14/07/21/mountain-4694346_960_720.png",
),
),
The image turns red. The output is given below.
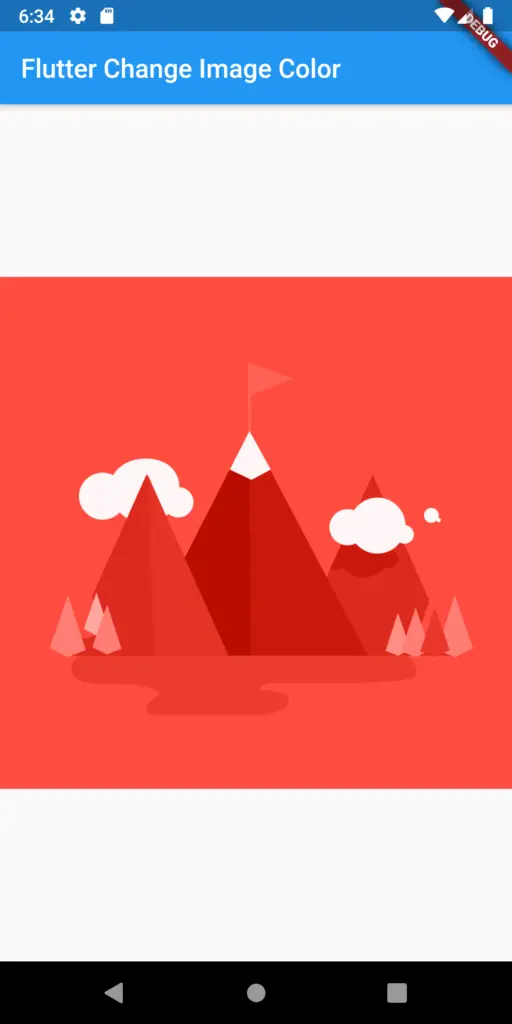
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Change Image Color'),
),
body: Center(
child: ColorFiltered(
colorFilter: const ColorFilter.mode(Colors.red, BlendMode.color),
child: Image.network(
"https://cdn.pixabay.com/photo/2019/12/14/07/21/mountain-4694346_960_720.png",
),
),
));
}
}
You can change the BlendMode to get different configurations while changing the color. Also, note that the ColorFiltered widget can be used to change the colors of widgets other than images too.
Before leaving, you may also check out – how to add borders around an image in Flutter.
I hope this Flutter Image color tutorial is helpful for you.