How to change Card Background Color in Flutter
The Card is one of the commonly used widgets in Flutter. In this blog post, let’s check how to change the default background color of the Card widget in Flutter.
Adding a Card in Flutter is pretty simple. The Card widget has many properties to customize it. The color property helps you to set the Card background color easily.
See the code snippet given below.
Card(
color: Colors.yellow,
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const ListTile(
leading: Icon(
Icons.abc,
size: 50,
),
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
TextButton(
child: const Text('GOT IT'),
onPressed: () {},
),
],
),
),
The output is given below.
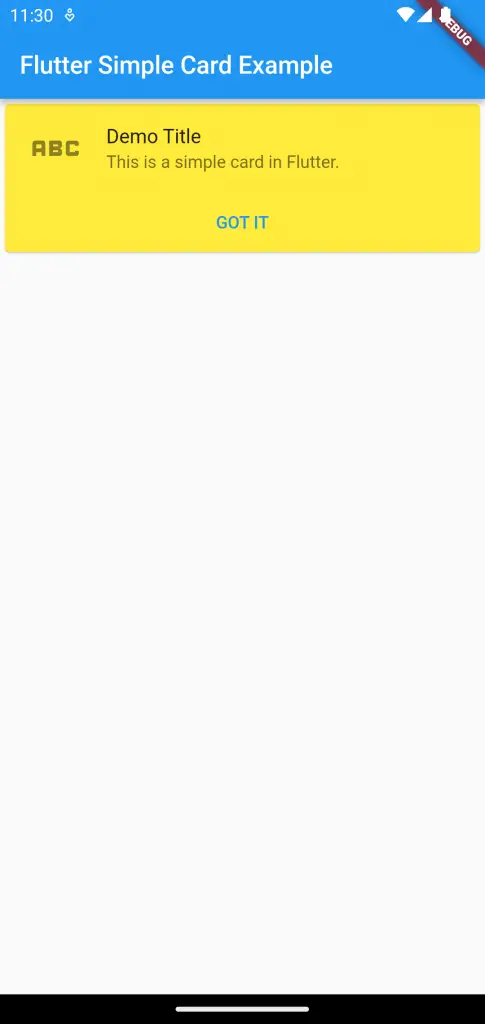
If you are using Material 3 then you will get the following output.
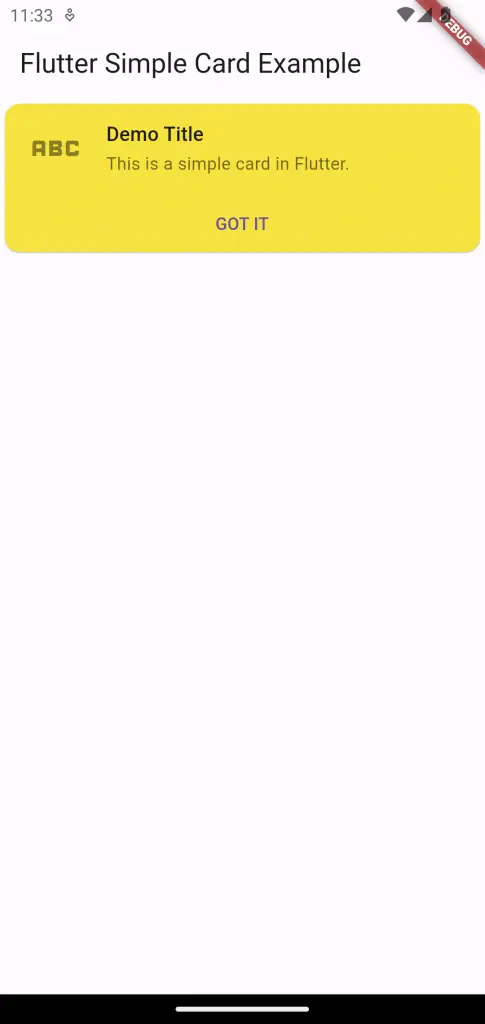
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// primarySwatch: Colors.blue,
colorSchemeSeed: const Color(0xff6750a4),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Simple Card Example'),
),
body: Card(
color: Colors.yellow,
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const ListTile(
leading: Icon(
Icons.abc,
size: 50,
),
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
TextButton(
child: const Text('GOT IT'),
onPressed: () {},
),
],
),
),
);
}
}
That’s how you change Card background color in Flutter.