How to Center Children Horizontally in Flutter Column
Let’s talk about centering children horizontally within a Column
widget. Even though Flutter is quite flexible in terms of layout options, knowing the precise way to align children horizontally within a column can be a bit tricky.
In this post, we’ll go over two different approaches to achieve this.
Approach 1: Use SizedBox and MediaQuery
The Code
SizedBox(
width: MediaQuery.of(context).size.width,
child: const Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text('First Child'),
Text('Second Child'),
],
)
)
Code Explanation
- SizedBox: The
SizedBox
widget helps you give a specific size to its child. In this case, we use it to set the width. - MediaQuery: The
MediaQuery
widget helps us to determine the screen width. We set theSizedBox
width to take up the entire screen width. - Column and CrossAxisAlignment: Inside the
SizedBox
, we add aColumn
and set itsCrossAxisAlignment
tocenter
to center the children horizontally.
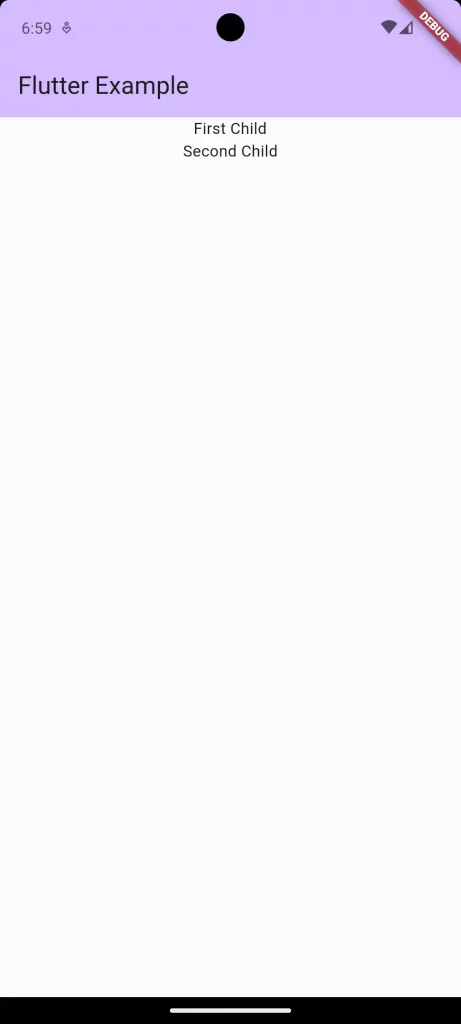
Approach 2: Use Container with Alignment
The Code
Container(
alignment: Alignment.center,
child: const Column(
children: [
Text('First Child'),
Text('Second Child'),
],
)
)
Code Explanation
- Container: We use a
Container
to wrap theColumn
. TheContainer
widget allows more freedom in terms of alignment and size. - Alignment: We set the
alignment
property of theContainer
toAlignment.center
. This aligns the childColumn
and its children to the center. - Column and Children: We place a
Column
inside theContainer
and add its children. These children will inherit the alignment from theContainer
.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
alignment: Alignment.center,
child: const Column(
children: [
Text('First Child'),
Text('Second Child'),
],
)));
}
}
Centering children horizontally within a Column
in Flutter is a task you can accomplish in multiple ways. You can either use a SizedBox
combined with MediaQuery
for fine-grained control or opt for a Container
with an alignment setting for a simpler approach.