How to Add Dropdown Menu in Flutter
Drop down menus are very useful ui components when you want a single input from multiple options. In this blog post, let’s check how to add a simple drop down menu in flutter.
DropdownButton class of flutter can be used to create a dropdown widget in flutter. It has important attributes such as value, items, onChanged etc. As the selected value needs to be changed everytime user picks an option, we must use a stateful widget here.
If you are looking for radio buttons, then you can checkout my blog post on adding radio buttons in flutter.
You can create a simple dropdown as given below.
DropdownButton(
value: selectedValue,
items: [
DropdownMenuItem(
child: Text("Male"),
value: 1,
),
DropdownMenuItem(
child: Text("Female"),
value: 2,
),
],
onChanged: (value) {
setState(() {
selectedValue = value;
});
})
Following is the complete example flutter dropdown menu.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Drop Down Menu Example',
home: DropDownExample(),
);
}
}
class DropDownExample extends StatefulWidget {
DropDownExample({Key key}) : super(key: key);
@override
_DropDownExampleState createState() => _DropDownExampleState();
}
class _DropDownExampleState extends State<DropDownExample> {
int selectedValue = 1;
@override
Widget build(BuildContext context) {
return Scaffold(
body:Container(
child: Padding(
padding: const EdgeInsets.all(10.0),
child:Center(
child: DropdownButton(
value: selectedValue,
items: [
DropdownMenuItem(
child: Text("Male"),
value: 1,
),
DropdownMenuItem(
child: Text("Female"),
value: 2,
),
DropdownMenuItem(
child: Text("Others"),
value: 3
),
],
onChanged: (value) {
setState(() {
selectedValue = value;
});
}),
)
),),);
}
}
Following is the output of the example.
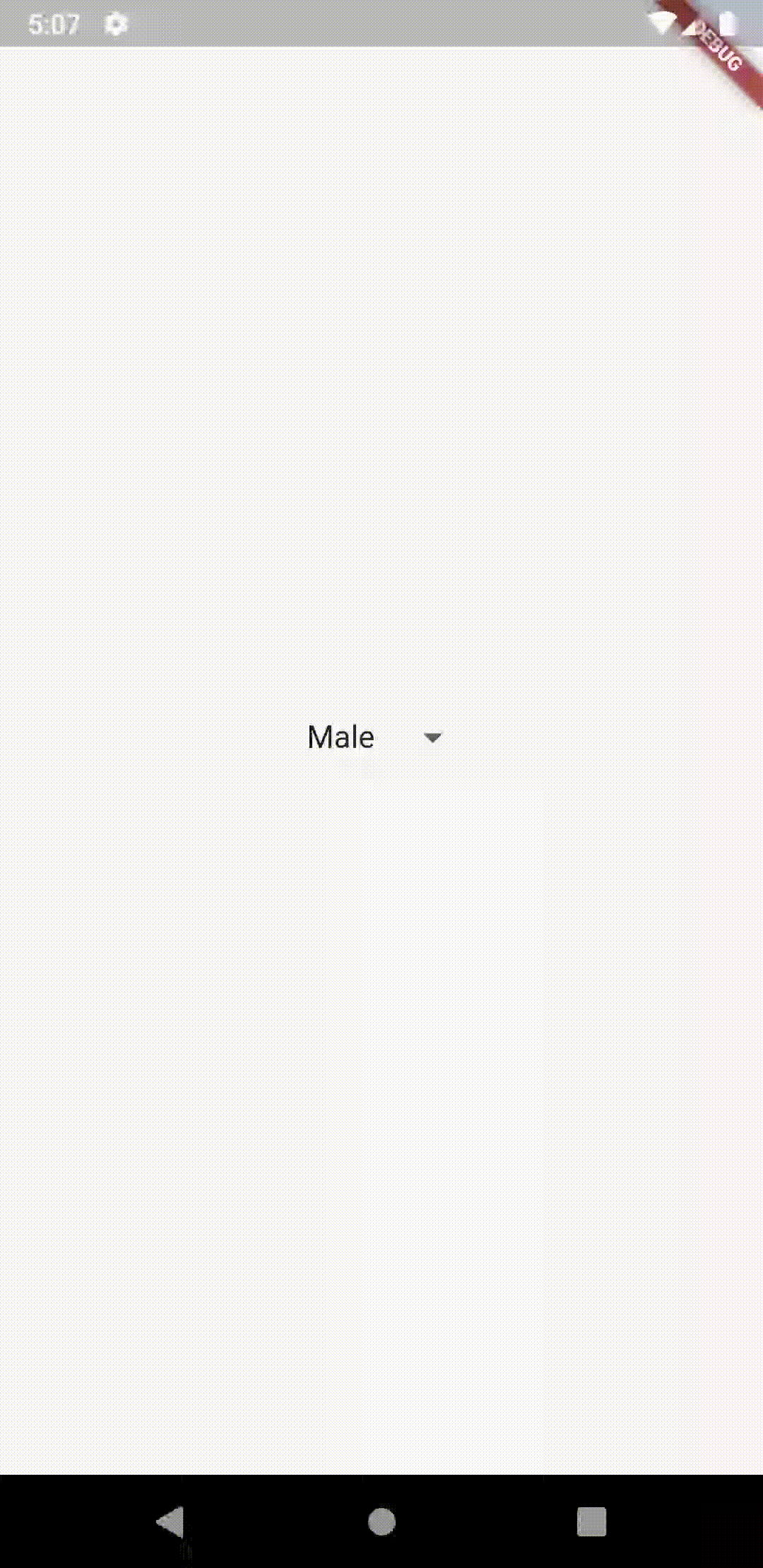
As I said earlier this is a simple drop-down menu. If you want to know more then go through the official documentation of DropdownButton widget.