How to Open a URL using WebView in Flutter
Sometimes, we may want to open a specific web page inside our mobile app so that users don’t feel they are opening a website. In such situations, a webview inside the app will be extremely useful. In this flutter tutorial, let’s learn how to open a URL using WebView in Flutter.
First of all, Flutter doesn’t have a dedicated inbuilt webview widget. Fortunately, there’s a plugin named webview flutter which is developed by the official flutter development team.
You can install the webview flutter using the following command.
flutter pub add webview_flutter
Make sure that minSdkVersion in android/app/build.gradle is 19 or greater than 19.
android {
defaultConfig {
minSdkVersion 19
...
}
}
Also, add internet permission in the Android Manifest file.
<uses-permission android:name="android.permission.INTERNET" />
You can import the package as given below.
import 'package:webview_flutter/webview_flutter.dart';
It has two parts: a WebViewController and a WebViewWidget. You have to initialize the WebViewController first.
late final WebViewController controller;
controller = WebViewController()
..setJavaScriptMode(JavaScriptMode.unrestricted)
..loadRequest(Uri.parse('https://flutter.dev'));
}
Then pass the controller to the WebViewWidget as given below.
WebViewWidget(controller: controller)
Following is the complete code to add WebView in Flutter.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
@override
void initState() {
super.initState();
controller = WebViewController()
..setJavaScriptMode(JavaScriptMode.unrestricted)
..loadRequest(Uri.parse('https://flutter.dev'));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView Example'),
),
body: WebViewWidget(controller: controller));
}
}
Following is the output on an Android device.
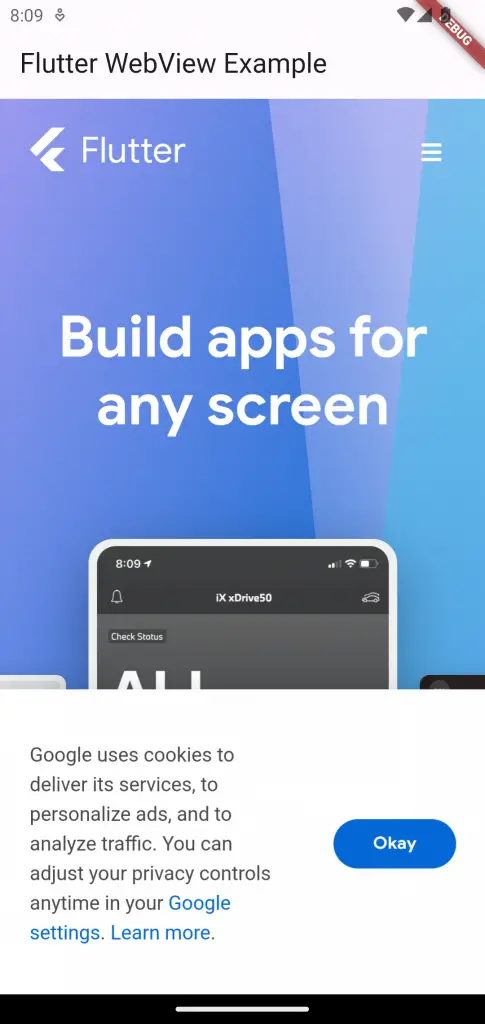
And following is the output in iOS.
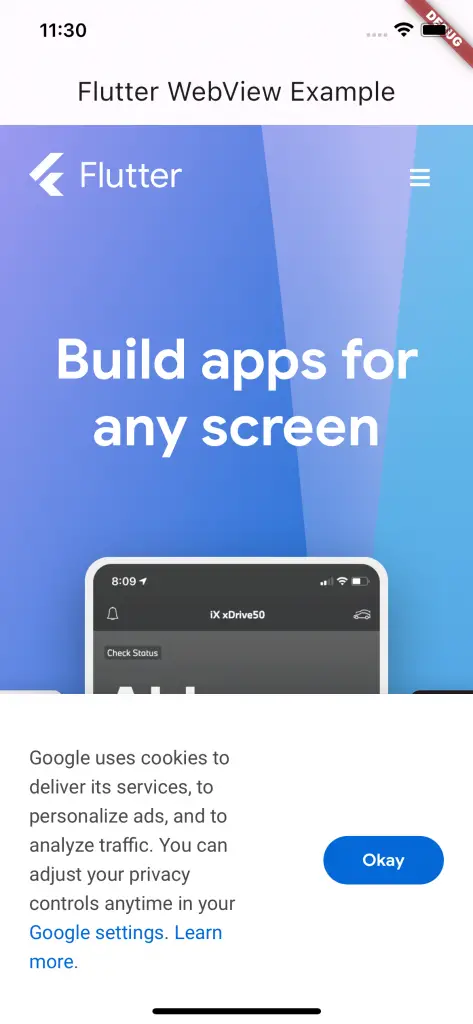
If you want to add a webview with progress indicator then see this tutorial.
That’s how you add a simple webview in Flutter. I hope this Flutter tutorial will be helpful for you.
3 Comments