How to Implement Row Alignment in Flutter
Let’s dive into one of the foundational aspects of building a great user interface in Flutter: aligning items in a Row
widget. Understanding alignment is crucial for creating a clean and visually appealing layout.
So, let’s explore different ways to achieve that perfect alignment in your Flutter Row
.
Basic Alignment Using mainAxisAlignment
The most straightforward way to control the alignment of items in a Row
is by using the mainAxisAlignment
property.
Sample Code
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(Icons.star),
Text('Hello, World!'),
Icon(Icons.star),
],
)
In the above code, mainAxisAlignment
is set to MainAxisAlignment.center
. This centers the items along the main axis, which is horizontal in a Row
.
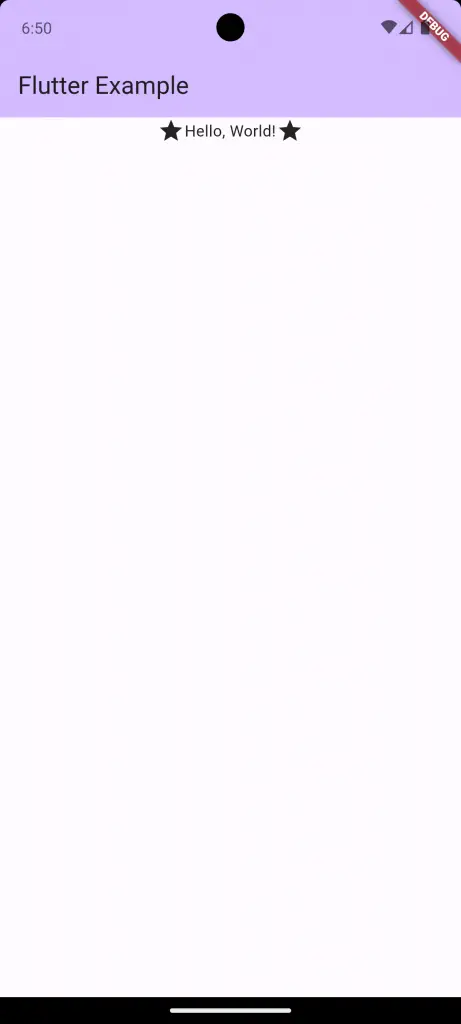
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(Icons.star),
Text('Hello, World!'),
Icon(Icons.star),
],
));
}
}
Understanding crossAxisAlignment
Another property to consider is crossAxisAlignment
, which aligns items perpendicular to the main axis.
Sample Code
const Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Icon(Icons.star),
Text('Hello, World!'),
Icon(Icons.star),
],
)
Setting crossAxisAlignment
to CrossAxisAlignment.start
aligns the items at the start of the row’s cross-axis, meaning they’ll be aligned at the top of the row.
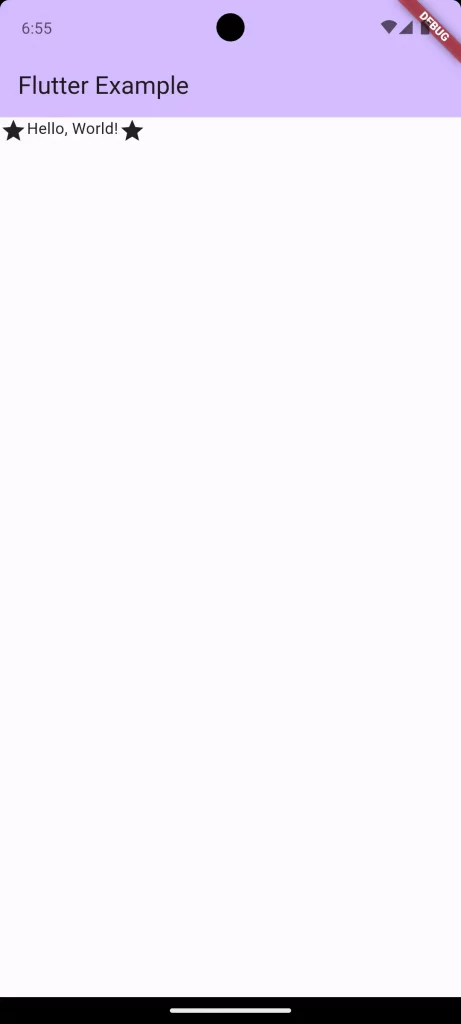
Use Spacer for Custom Alignment
For more fine-grained control over alignment, the Spacer
widget is invaluable.
Sample Code
const Row(
children: [
Icon(Icons.star),
Spacer(flex: 1),
Text('Hello, World!'),
Spacer(flex: 2),
Icon(Icons.star),
],
)
The Spacer
widget takes up available space within a Row
, pushing adjacent widgets accordingly. The flex
property defines how much space each Spacer
should occupy relative to others.
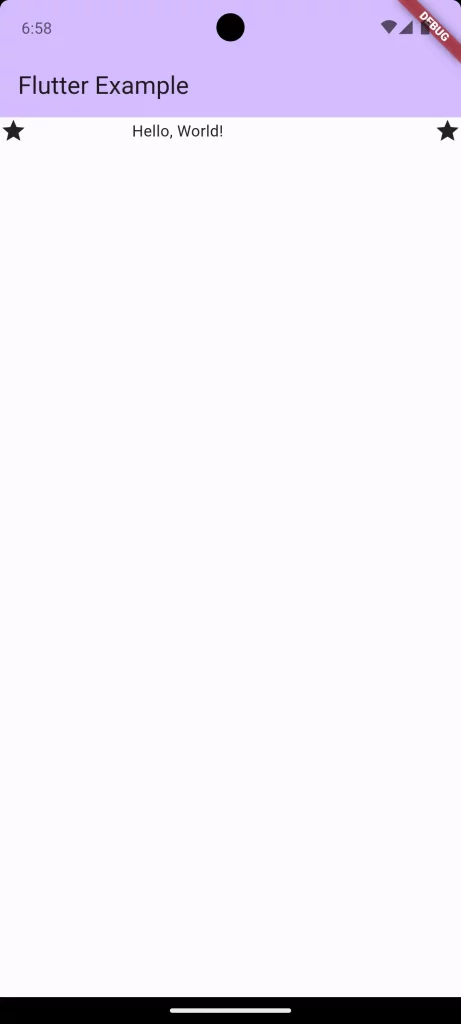
Flutter offers a wealth of options for aligning items in a Row
, from simple properties like mainAxisAlignment
and crossAxisAlignment
to more advanced widgets like Spacer
. Understanding these different methods will give you greater control over your app’s layout, ensuring you can align items in a Row
just the way you want.