How to Change TextField Placeholder Color in iOS SwiftUI
A well-designed user interface often requires customization, even down to small details like the color of a placeholder text. In SwiftUI, changing the placeholder color of a TextField
is not straightforward.
In this blog post, we’ll explore a simple way to customize the placeholder color of a TextField
using SwiftUI.
In this tutorial, we will make use of the prompt
modifier.
Here’s an example of how to achieve this customization:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("", text: $text, prompt: Text("Placeholder").foregroundColor(Color.orange))
.padding()
}
}
In the above code snippet, the prompt
modifier accepts a Text
view that allows you to define the placeholder string. You can then apply the foregroundColor
modifier to change its color.
This code will produce a text field with an orange-colored placeholder text saying “Placeholder.” When the user starts typing, the placeholder will disappear, just like any standard text field.
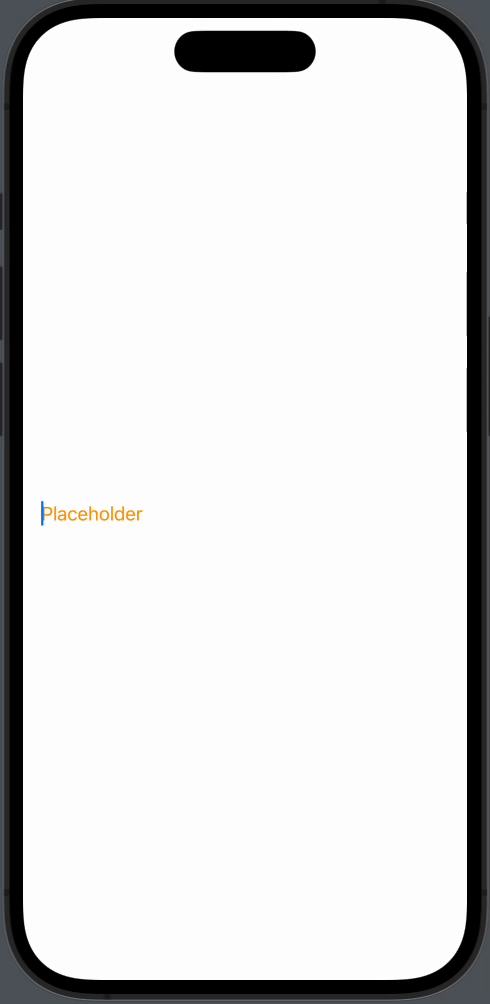
Customizing the placeholder color of a TextField
in SwiftUI can be done elegantly using the prompt
modifier. This technique provides a simple and native way to tailor your app’s appearance, adding to the overall user experience.