How to change AppBar Height in Flutter
AppBar is one of the most important widgets in Flutter. It helps developers to set a sticky toolbar at the top of each screen. Let’s check how to change the default height of AppBar in Flutter.
Changing the height of AppBar is easy. You just need to use toolbarHeight property of AppBar. See the code snippet given below.
AppBar(
toolbarHeight: 100,
title: const Text(
'Flutter AppBar Height',
),
),
You will get the following output.
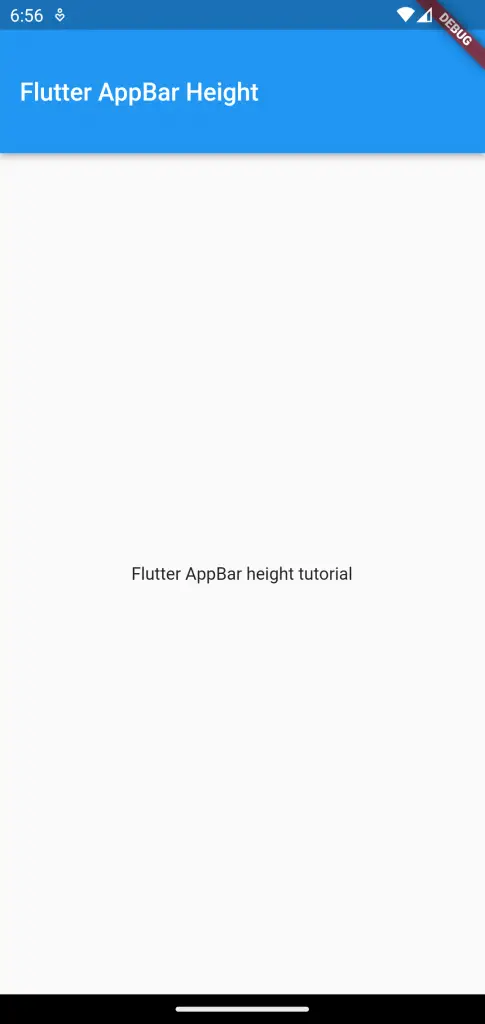
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
toolbarHeight: 100,
title: const Text(
'Flutter AppBar Height',
),
),
body: const Center(child: Text('Flutter AppBar height tutorial')));
}
}
I hope this Flutter AppBar height tutorial is helpful for you.