How to Center Align a Column in Flutter
If you’re a Flutter developer, you must have faced the common challenge of aligning widgets on the screen. In this blog post, I’m going to discuss two methods for center aligning a column in Flutter.
Method 1: Using SizedBox and MediaQuery
In this method, we align the children to the center of the column.
The Code
Here’s the example code using SizedBox
and MediaQuery
:
SizedBox(
width: MediaQuery.of(context).size.width,
child: const Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text('First Child'),
Text('Second Child'),
],
)
)
Code Explanation
- SizedBox: The
SizedBox
widget is used to give a specific size to its child widget. Here, we specify the width usingMediaQuery
. - MediaQuery: We use
MediaQuery.of(context).size.width
to dynamically get the screen width. This ensures that the column takes up the entire width of the screen. - Column: Inside the
SizedBox
, we place aColumn
widget withmainAxisAlignment
andcrossAxisAlignment
set tocenter
. This makes sure that the children are centered.
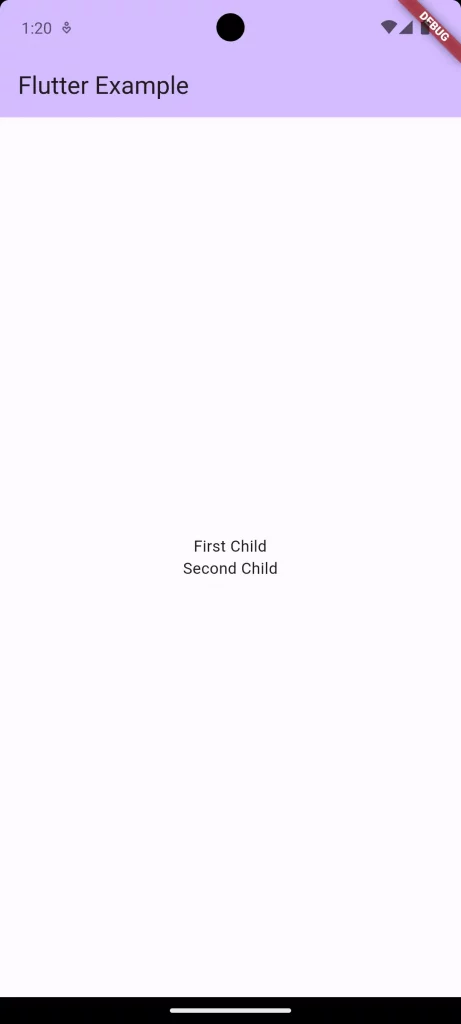
Method 2: Using Center Widget
In this method, we align the column itself to the center.
The Code
Here’s the alternative way to center align a column using the Center
widget:
const Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text('First Child'),
Text('Second Child'),
],
)
)
Code Explanation
- Center Widget: The
Center
widget automatically aligns its child to the center of the parent widget. - Column: Just like in the first example, we use a
Column
withmainAxisAlignment
andcrossAxisAlignment
set tocenter
.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text('First Child'),
Text('Second Child'),
],
)));
}
}
Both methods have their pros and cons, but they effectively achieve the same goal: center aligning a column in Flutter. The Center
widget is easier to use, while the SizedBox
and MediaQuery
offer more control. Pick the one that suits your needs the best!