How to set ListView Background Color in Flutter
ListView is one of the most used widgets in Flutter. It helps to list a finite or infinite set of items quickly. In this blog post, let’s check how to change the background color of ListView in Flutter.
The ListView widget doesn’t have a property to change the background color. Hence, we may wrap ListView with a Container widget to change the color. The color property of the Container helps to set the color.
See the code snippet given below.
Container(
color: Colors.grey,
child: ListView.builder(
itemCount: data.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.yellow,
margin: const EdgeInsets.all(8),
child: Center(child: Text(data[index])),
);
})
Here, the background color is grey, and the background color of each ListView item is yellow.
See the following output.
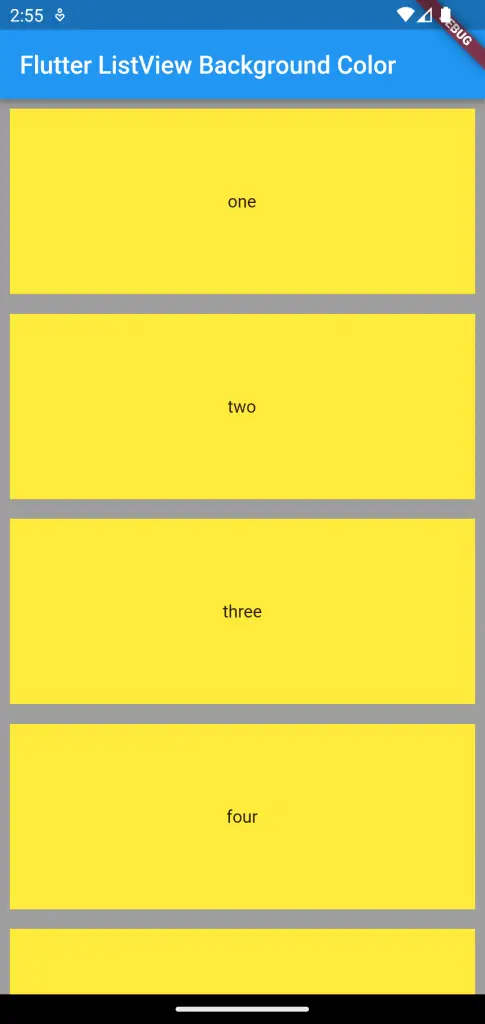
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
final data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter ListView Background Color',
),
),
body: Container(
color: Colors.grey,
child: ListView.builder(
itemCount: data.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.yellow,
margin: const EdgeInsets.all(8),
child: Center(child: Text(data[index])),
);
})));
}
}
That’s how you set the ListView background color in Flutter.