How to Set Background Image in Flutter Column
In this blog post, we are going to talk about an interesting topic that can take your UI game to the next level—setting a background image in a Column
widget in Flutter.
Flutter doesn’t provide a direct backgroundImage
property on the Column
widget, but there are workarounds to achieve this effect.
Use BoxDecoration with an Image
What is BoxDecoration?
BoxDecoration
is a widget in Flutter used to paint a decoration on a Container
. It has a property called image
which can be set to create background images.
The Code
Here’s a simple code snippet to set a background image to a Column
using BoxDecoration
:
Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.jpg"),
fit: BoxFit.cover,
),
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Text('First Child'),
Text('Second Child'),
],
),
)
Code Explanation
- Container Widget: The
Container
widget wraps ourColumn
. - BoxDecoration: Inside the
Container
, we use theBoxDecoration
class. - DecorationImage: Within
BoxDecoration
, we set theimage
property usingDecorationImage
. - AssetImage: We specify the path to the image asset we want to use.
- BoxFit.cover: This makes sure the image covers the entire container while maintaining its aspect ratio.
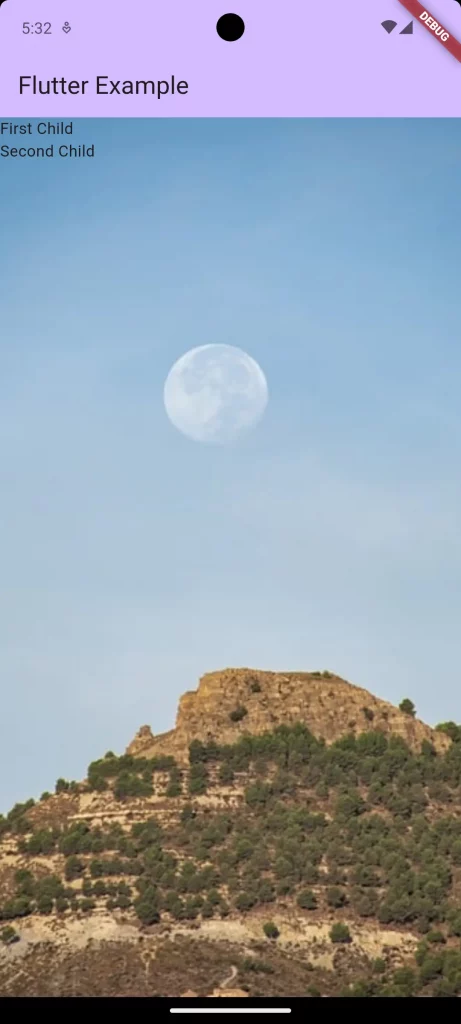
Use Stack for More Complex Layouts
What is Stack?
A Stack
allows you to overlay multiple children on top of each other. You can use it to place a Column
over an Image
widget.
The Code
Stack(
children: [
Image.asset("assets/background.jpg", fit: BoxFit.cover),
Column(
children: [
Text('First Child'),
Text('Second Child'),
],
),
],
)
Code Explanation
- Stack Widget: We use
Stack
to overlay widgets. - Image.asset: The background image is set by using the
Image.asset
widget. - Column Widget: The
Column
widget is placed on top of the image.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.jpg"),
fit: BoxFit.cover,
),
),
child: Stack(
children: [
Image.asset("assets/background.jpg", fit: BoxFit.cover),
const Column(
children: [
Text('First Child'),
Text('Second Child'),
],
),
],
)));
}
}
Setting a background image in a Column
in Flutter can be achieved in a couple of ways, depending on your needs. The BoxDecoration
method is more straightforward for simpler layouts, while using a Stack
allows for greater flexibility in more complex UIs.