How to Open PDF in Flutter using PDF Viewer
PDF files are an integral part of our daily lives and we often come across a situation where we need to view PDF files on our mobile devices. In this blog post, let’s learn how to prompt the flutter app to open a PDF file using the default PDF viewer of your Android or iOS phone.
We use open_filex package for this tutorial. The open_filex package can call native apps to open files in Flutter.
Install the package using the following command.
flutter pub add open_filex
You can use the library as given below.
import 'package:open_filex/open_filex.dart';
OpenFilex.open("/sdcard/example.pdf");
Following is the code to open a pdf file using the inbuilt pdf viewer.
import 'package:flutter/material.dart';
import 'package:open_filex/open_filex.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(useMaterial3: true),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Pdf Example',
),
),
body: Center(
child: ElevatedButton(
style: ElevatedButton.styleFrom(elevation: 10),
onPressed: () {
OpenFilex.open("/storage/emulated/0/Download/sample.pdf");
},
child: const Text('Elevated Button'))));
}
}
Here, when the elevated button is pressed, the pdf file from the given path will be opened.
Following is the output.
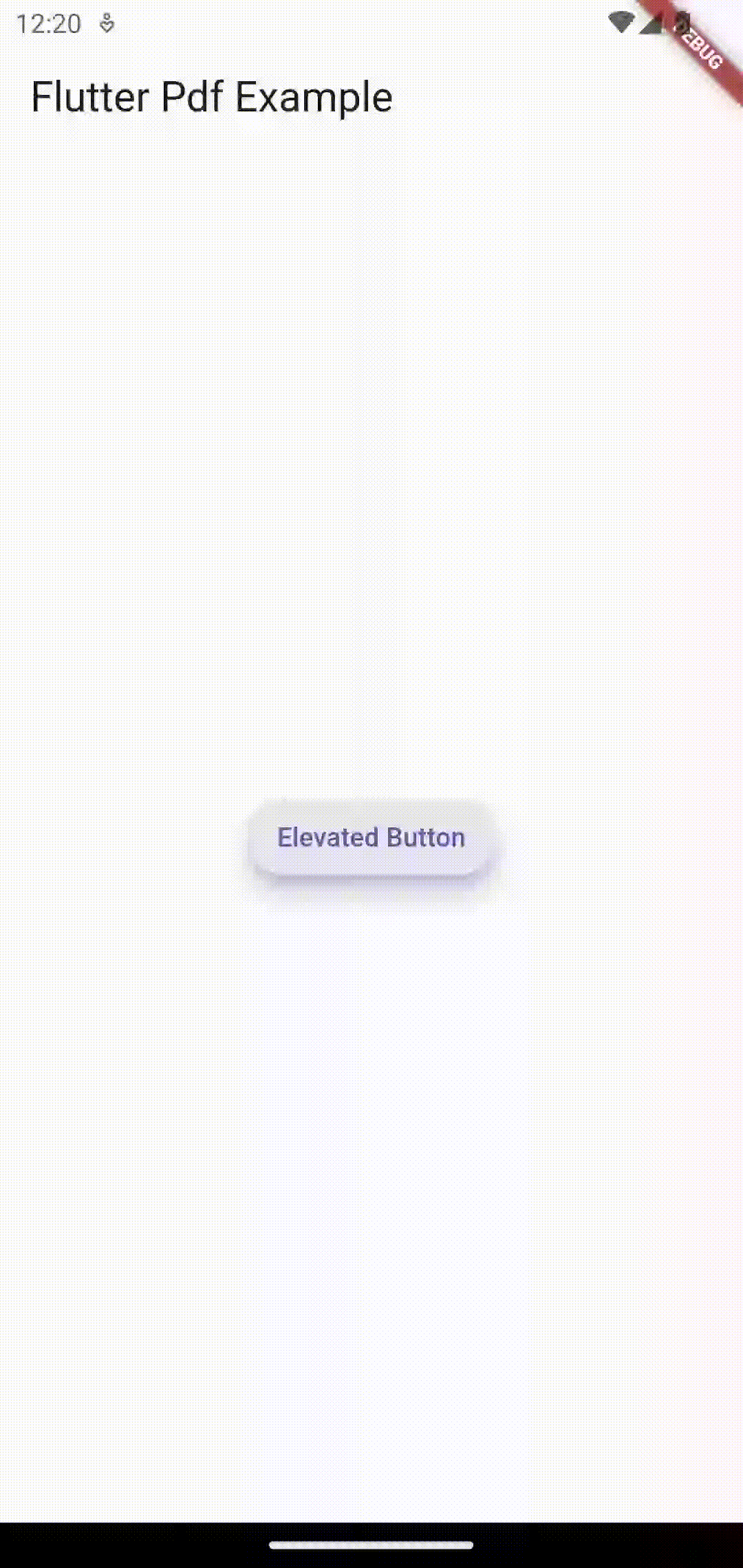
That’s how you open pdf files easily in Flutter.
If you want to download the pdf file from a URL then see this tutorial. After downloading, you can use the open_filex package to open it.
You can also use other packages to open PDF file within the Flutter app.