How to Make TextInput Number Only in React Native
Collecting numerical input is a common requirement for mobile apps. React Native’s TextInput
component makes this task easier but doesn’t restrict the input to numbers by default.
This blog post will explain how to make TextInput
accept only numeric input, covering various methods and providing practical examples.
Prerequisites
- A functional React Native environment
- Basic understanding of React Native and JavaScript
Basic Usage of TextInput
Let’s start by looking at how to create a simple TextInput
component.
import React from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
return (
<View>
<TextInput placeholder="Type anything here" />
</View>
);
};
Use keyboardType
for Numeric Input
The easiest way to restrict input to numbers is by setting the keyboardType
prop to “numeric”, “decimal-pad
” or “phone-pad
“.
<TextInput keyboardType="numeric" placeholder="Type numbers here" />
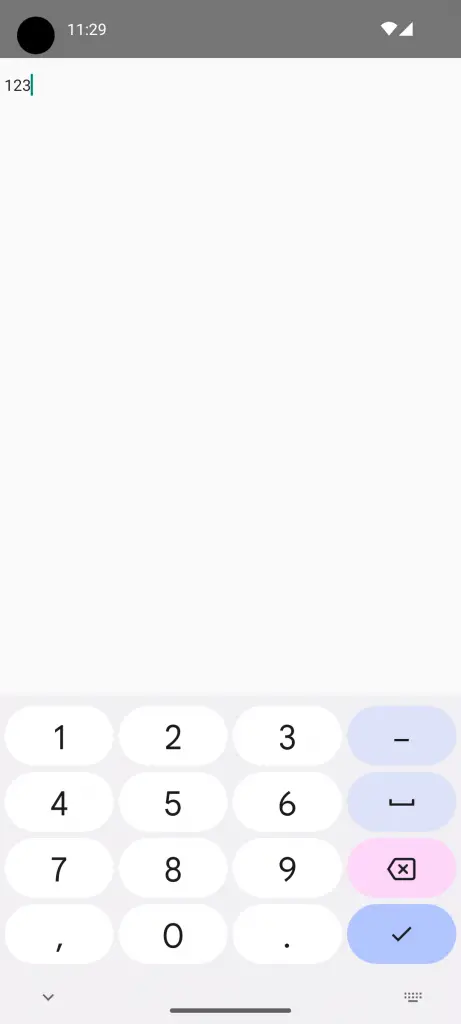
You can see screenshots all keyboard types here.
Restricting a TextInput
to only accept numeric input is an essential feature for various scenarios like collecting phone numbers or amounts. By setting the keyboardType
prop you can ensure that the input field is as fool-proof as possible.