How to Integrate Mapbox in React Native
Mapbox is considered as one of the best alternatives for Google Maps. If you are looking for a Google Maps alternative for your next react native project then you are at the right place. In this react native mapbox tutorial, I am going to create a simple project where maps are shown using mapbox with some cordinates marked.
Mapbox has a generous free tier where you can have upto 25,000 monthly active users. You also don’t need to connect a credit card with for creating an account with Mapbox, unlike in the case of Google Maps.
First of all, you need to get an access token from Mapbox. It’s pretty easy, go here and create an account. Then go to your Mapbox account and create an access token for your project.
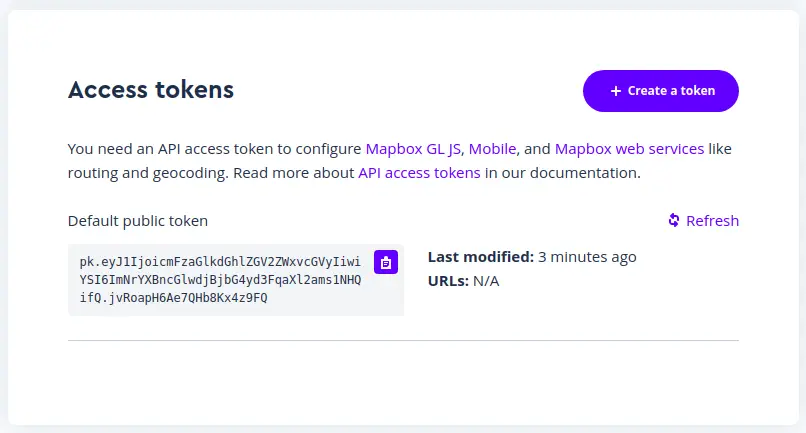
I hope you already have a react native project to work with. The official react native library of the Mapbox team is deprecated. Hence, we use the community-driven library react native mapbox gl/maps.
Install the library using any of the commands below.
yarn add @react-native-mapbox-gl/maps
or
npm install @react-native-mapbox-gl/maps –save
If you are on iOS then don’t forget to run pod install.
Once the initial set up is ready, we can start doing the coding part.
You can import MapboxGl component from the library as given below.
import MapboxGL from '@react-native-mapbox-gl/maps';
You have to initialize Mapbox using the token you generated from their website as given below.
MapboxGL.setAccessToken(
'pk.eyJ1IjoicmFzaGlkdGhlZGV2ZWxvcGVyIiwiYSI6ImNrYXBncGlwdjBjbG4yd3FqaXl2ams1NHQifQ.jvRoapH6Ae7QHb8Kx4z9F'
);
We use three components namely MapboxGL.MapView, MapboxGL.Camera and MapboxGL.PointAnnotation. MapboxGL.MapView is used to show the map whereas MapboxGL.Camera helps to show a particular area in the beginning. MapboxGL.PointAnnotation is used to annotate a location with the given latitude and longitude of a location.
Following is the complete code show a maps with a marker at a given latitude and longitude.
import React, {Component} from 'react';
import {StyleSheet, View} from 'react-native';
import MapboxGL from '@react-native-mapbox-gl/maps';
MapboxGL.setAccessToken(
'pk.eyJ1IjoicmFzaGlkdGhlZGV2ZWxvcGVyIiwiYSI6ImNrYXBncGlwdjBjbG4yd3FqaXl2ams1NHQifQ.jvRoapH6Ae7QHb8Kx4z9FQ',
);
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
coordinates: [-73.99155, 40.73581],
};
}
render() {
return (
<View style={styles.page}>
<View style={styles.container}>
<MapboxGL.MapView style={styles.map}>
<MapboxGL.Camera
zoomLevel={8}
centerCoordinate={this.state.coordinates}
/>
<MapboxGL.PointAnnotation coordinate={this.state.coordinates} />
</MapboxGL.MapView>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
page: {
flex: 1,
},
container: {
height: '100%',
width: '100%',
backgroundColor: 'blue',
},
map: {
flex: 1,
},
});
If you are a fan of react hooks then choose the code given below.
import React, {useState} from 'react';
import {StyleSheet, View} from 'react-native';
import MapboxGL from '@react-native-mapbox-gl/maps';
MapboxGL.setAccessToken(
'pk.eyJ1IjoicmFzaGlkdGhlZGV2ZWxvcGVyIiwiYSI6ImNrYXBncGlwdjBjbG4yd3FqaXl2ams1NHQifQ.jvRoapH6Ae7QHb8Kx4z9FQ',
);
const App = () => {
const [coordinates] = useState([-73.99155, 40.73581]);
return (
<View style={styles.page}>
<View style={styles.container}>
<MapboxGL.MapView style={styles.map}>
<MapboxGL.Camera zoomLevel={8} centerCoordinate={coordinates} />
<MapboxGL.PointAnnotation coordinate={coordinates} id="Test" />
</MapboxGL.MapView>
</View>
</View>
);
};
const styles = StyleSheet.create({
page: {
flex: 1,
},
container: {
height: '100%',
width: '100%',
backgroundColor: 'blue',
},
map: {
flex: 1,
},
});
export default App;
The output of this react native mapbox example is as given below.

As you see, this react native mapbox tutorial is pretty basic. Mapbox offers so many features. If you want to use them then please go through their library.
Hi, Rashid. I want the map to use all the screen as it is supposed to be in your example, but if I set width and height to ‘100%’ it just displays a white screen
Hi, Rashid. I want the map to use all the screen as it is supposed to be in your example, but if I set width and height to ‘100%’ it just displays a white screen