How to Hide TextInput Cursor in React Native
The cursor is a crucial part of any text input field, guiding the user while typing. However, there are specific scenarios where hiding the cursor may be beneficial. This blog post will guide you on how to hide the cursor in a React Native TextInput
component.
Prerequisites
- A working React Native development environment
- Basic familiarity with React Native and JavaScript
Basic TextInput Implementation
Let’s start by understanding how a basic TextInput
field is created in React Native.
import React from 'react';
import { View, TextInput } from 'react-native';
const App = () => {
return (
<View>
<TextInput placeholder="Default cursor here" />
</View>
);
};
export default App;
Hide the Cursor Using the caretHidden
Prop
React Native offers the caretHidden
prop to control the visibility of the cursor.
<TextInput placeholder="No cursor here" caretHidden={true} />
Setting the caretHidden
prop to true
will hide the cursor when the TextInput
is focused.
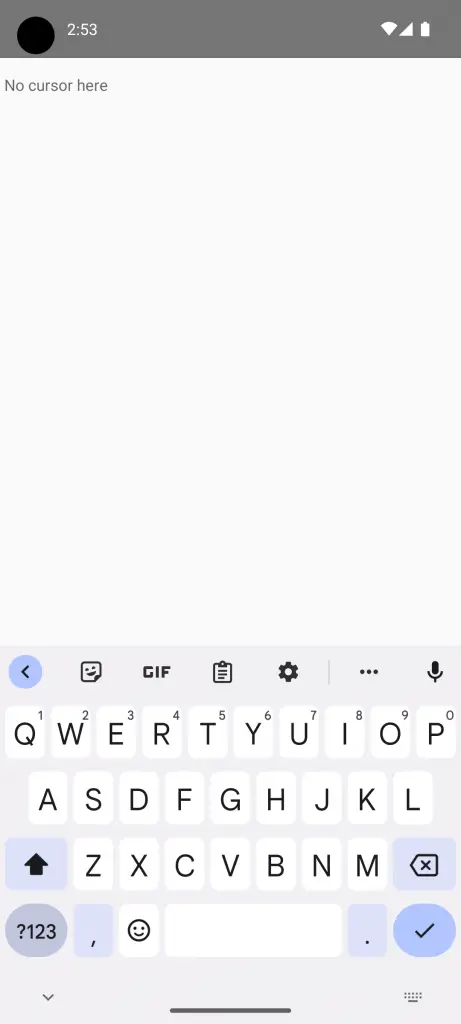
Conditional Hiding of Cursor
You may also need to hide the cursor under specific conditions, like when a maximum character limit is reached.
import React from 'react';
import {View, TextInput} from 'react-native';
const App = () => {
const [text, setText] = React.useState('');
return (
<View>
<TextInput
placeholder="Max 10 characters"
maxLength={10}
onChangeText={input => setText(input)}
caretHidden={text.length >= 10}
/>
</View>
);
};
export default App;
The maxLength
prop limits text input to a certain length. The cursor disappears when the maximum limit is reached.
Hiding the cursor in a TextInput
field in React Native is a simple but effective way to guide user behavior. Whether you want to hide it by default or under specific conditions, React Native provides the caretHidden
prop for easy implementation.