How to Create a Button with Ripple Effect in React Native
In this blog post, I will show you how to create a button with a ripple effect in react native without using any external library. We all know that ripple effects come with material design. The ripple effect helps the user to identify that his action such as a button press is registered.
We use the Pressable component to create the button. We use the android_ripple property of the Pressable so that we can create a ripple effect in the Android platform. If you are not familiar with the Pressable component then you can check out my blog post on how to use Pressable component in react native.
The android_ripple prop accepts an object where we can customize ripple color and radius. You can check the official docs here.
Following is an example of a button with a ripple effect in react native.
import React from 'react';
import {StyleSheet, Pressable, Text, View} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Pressable
style={styles.button}
onPress={() => console.log('pressed')}
android_ripple={{color: 'green'}}>
<Text style={styles.buttonText}>Button</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: 'white',
justifyContent: 'center',
alignItems: 'center',
},
button: {
borderRadius: 8,
padding: 6,
height: 50,
width: '70%',
justifyContent: 'center',
alignItems: 'center',
elevation: 5,
backgroundColor: 'cyan',
},
buttonText: {
fontSize: 16,
color: 'black',
},
});
export default App;
The example creates a green color ripple effect when the button is pressed. See the output given below.
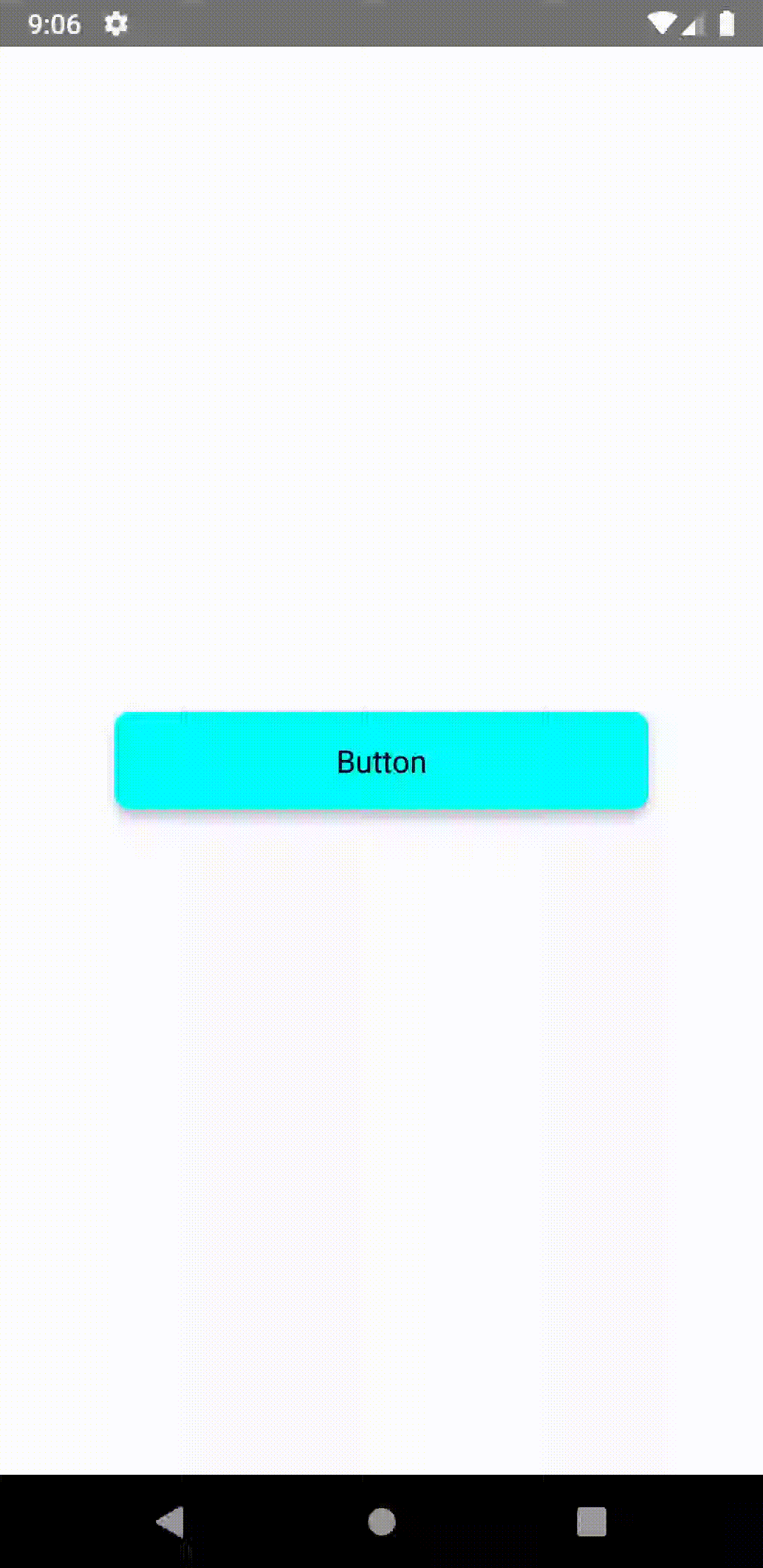
I hope this react native tutorial will help you.
How can I get ripple effect on an image? Using pressable, the ripple effect does not occur over the image. Any thoughts?
How can I get ripple effect on an image? Using pressable, the ripple effect does not occur over the image. Any thoughts?