How to Add Space Between Row Items in Flutter
One of the aspects of Flutter that I absolutely love is its flexibility in layout design. When it comes to arranging items in a row, Flutter offers powerful options for controlling the spacing between widgets.
In this blog post, we’ll deep dive into how to manage space between items in a Flutter Row
.
The mainAxisAlignment Property
The mainAxisAlignment
property is the most straightforward way to control spacing in a Row
. This property offers a variety of options, including spaceBetween
, spaceAround
, and spaceEvenly
.
spaceBetween
const Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Icon(Icons.home),
Icon(Icons.search),
Icon(Icons.person),
],
)
With spaceBetween
, the first and last items align to the edges of the Row
, and the remaining space is distributed evenly between the other items.
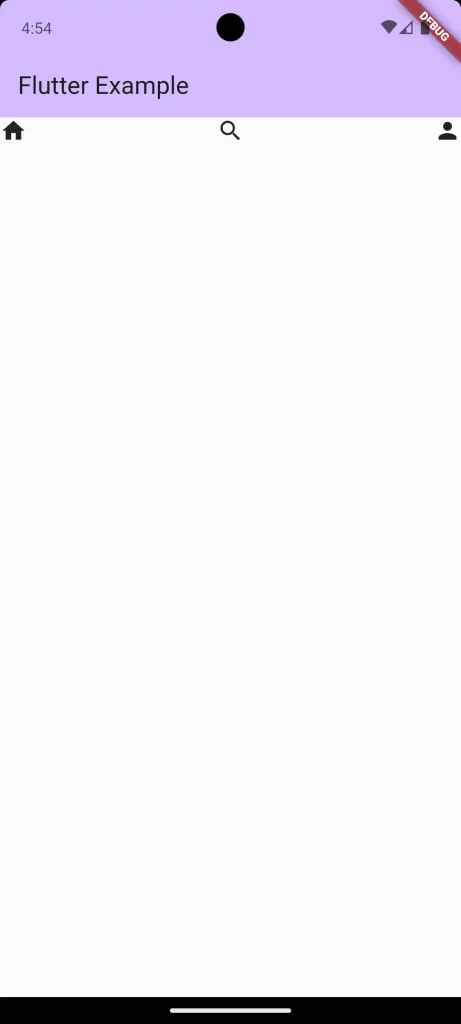
spaceAround
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Icon(Icons.home),
Icon(Icons.search),
Icon(Icons.person),
],
)
spaceAround
also distributes the space evenly, but unlike spaceBetween
, it allocates half of the space before the first item and after the last item.
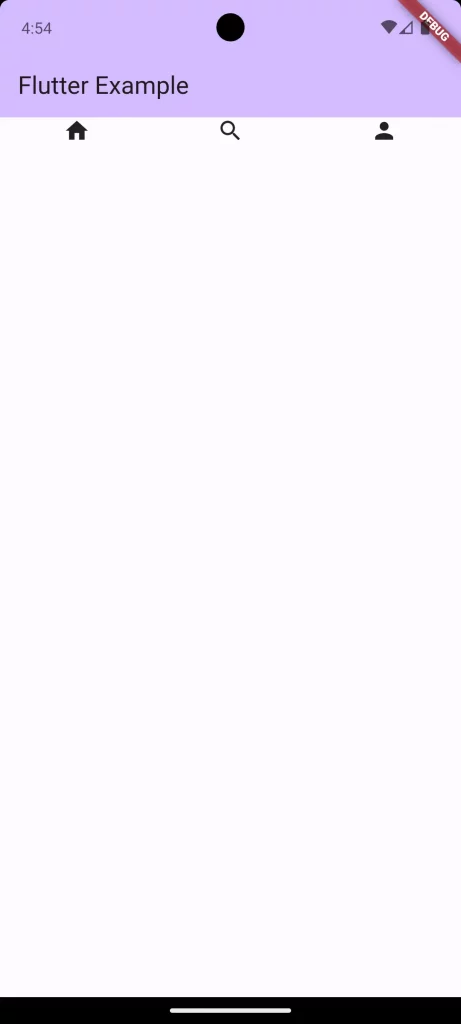
spaceEvenly
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Icon(Icons.home),
Icon(Icons.search),
Icon(Icons.person),
],
)
spaceEvenly
divides the space evenly between all the items, including the first and last items, creating an even amount of space between each widget.
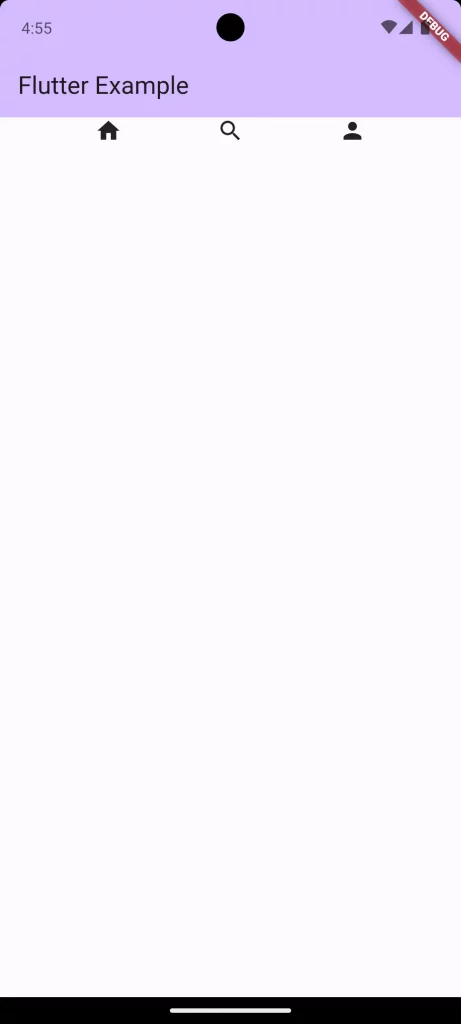
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Icon(Icons.home),
Icon(Icons.search),
Icon(Icons.person),
],
));
}
}
Managing space between items in a Flutter Row
is easy yet powerful, thanks to the mainAxisAlignment
property. Whether you choose spaceBetween
, spaceAround
, or spaceEvenly
, each option has its own set of advantages that can make your application’s layout cleaner and more organized.