How to add Radio Button with Text in Flutter
The radio button is a useful UI component to choose a single value from multiple options. In this blog post, let’s learn how to add a radio button with a label in Flutter.
There are multiple ways to add a radio with text in Flutter. Here, we choose RadioListTile class to create a radio button with a label. It is basically a radio button on a tile. The radio button toggles even when the user clicks anywhere on the tile.
See the code snippet given below.
RadioListTile<Animals>(
title: const Text('Giraffe'),
value: Animals.giraffe,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
)
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Radio Button with Label'),
),
body: const MyStatefulWidget());
}
}
enum Animals { giraffe, lion }
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
Animals? _animal = Animals.giraffe;
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
RadioListTile<Animals>(
title: const Text('Giraffe'),
value: Animals.giraffe,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
),
RadioListTile<Animals>(
title: const Text('Lion'),
value: Animals.lion,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
),
],
);
}
}
And you will get the following output.
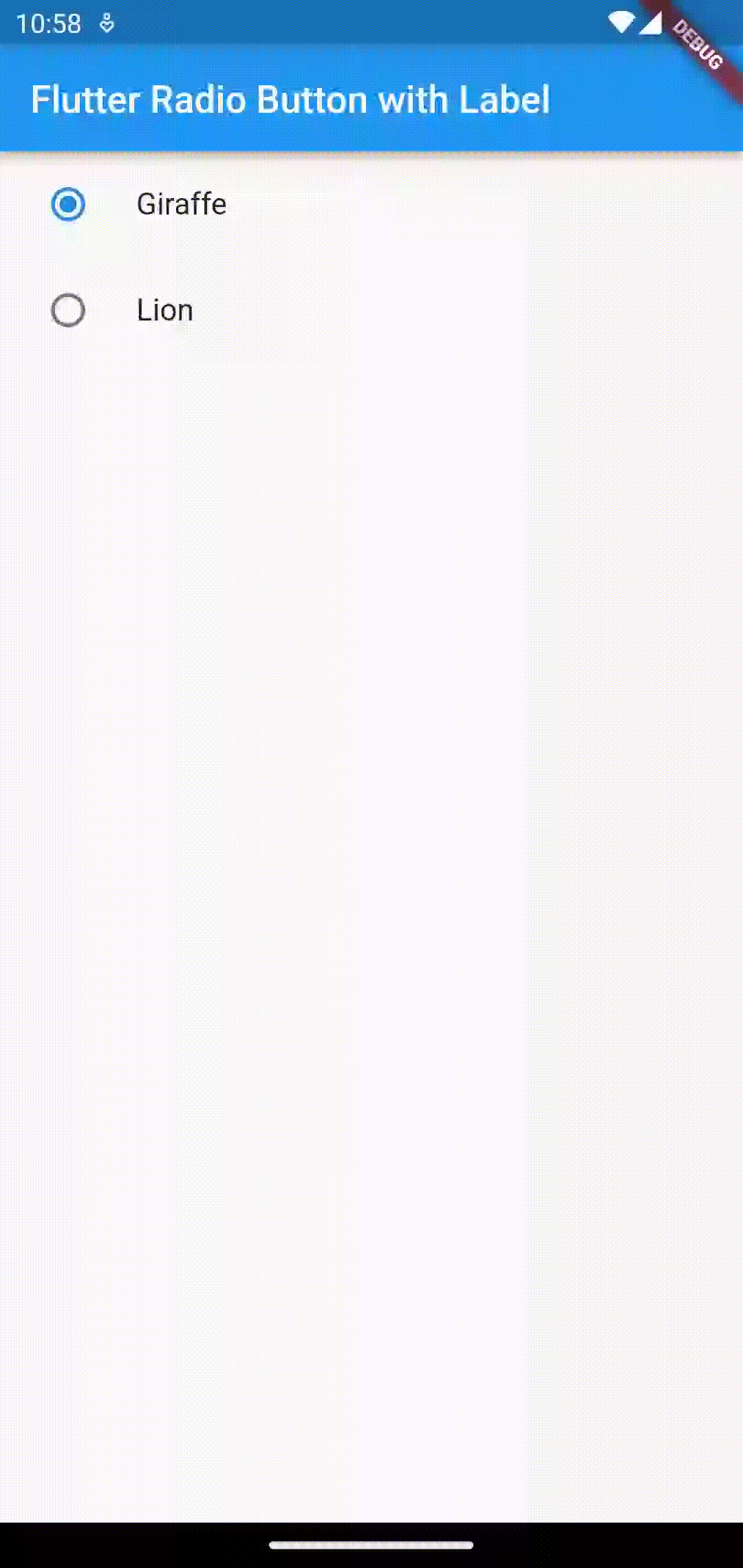
That’s how you add a radio button with text in Flutter.