How to Drag and Reorder FlatList Items in React Native
FlatList is one of the most used components in react native. It helps to render a list of items smoothly. Having a drag and reorder feature in FlatList helps users to arrange the items as they wish. This feature is very useful when the user wants to change the priority of items in the list.
Creating a drag and reorder feature with smooth animation from scratch can be a tedious task. Hence, in this react native example, I am showing you how to drag and reorder items in a FlatList using a third-party library named react native draggable flatlist.
React native draggable list uses two libraries for animation- react native animated and react native gesture handler. Install these libraries first by following the instructions from their pages and then proceed to install react native draggable list using any of the commands given below.
npm install --save react-native-draggable-flatlist
or
yarn add react-native-draggable-flatlist
After installation, you have to import DraggableFlatList and ScaleDecorator. You can also choose other components such as ShadowDecorator and OpacityDecorator. The ScaleDecorator scales up the selected item which is about to drag.
import DraggableFlatList, {
ScaleDecorator,
} from 'react-native-draggable-flatlist';
The usage of DraggableFlatList is very similar to FlatList. The onDragEnd prop helps to reorder the data.
<DraggableFlatList
data={data}
onDragEnd={({data}) => setData(data)}
keyExtractor={item => item.id}
renderItem={renderItem}
/>
Wrap the renderItem with ScaleDecorator.
const renderItem = ({item, drag, isActive}) => (
<ScaleDecorator>
<TouchableOpacity
onLongPress={drag}
disabled={isActive}
style={styles.item}>
<Text style={styles.title}>{item.title}</Text>
</TouchableOpacity>
</ScaleDecorator>
);
Following is the complete code.
import React, {useState} from 'react';
import {View, StyleSheet, TouchableOpacity, Text} from 'react-native';
import DraggableFlatList, {
ScaleDecorator,
} from 'react-native-draggable-flatlist';
const App = () => {
const [data, setData] = useState([
{
id: 'c1b1-46c2-aed5-3ad53abb28ba',
title: 'First Item',
},
{
id: 'c605-48d3-a4f8-fbd91aa97f63',
title: 'Second Item',
},
{
id: '3da1-471f-bd96-145571e29d72',
title: 'Third Item',
},
]);
const renderItem = ({item, drag, isActive}) => (
<ScaleDecorator>
<TouchableOpacity
onLongPress={drag}
disabled={isActive}
style={styles.item}>
<Text style={styles.title}>{item.title}</Text>
</TouchableOpacity>
</ScaleDecorator>
);
return (
<View>
<DraggableFlatList
data={data}
onDragEnd={({data}) => setData(data)}
keyExtractor={item => item.id}
renderItem={renderItem}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
item: {
backgroundColor: '#f9c2ff',
padding: 20,
marginVertical: 8,
marginHorizontal: 16,
},
title: {
fontSize: 32,
},
});
export default App;
And following is the output.
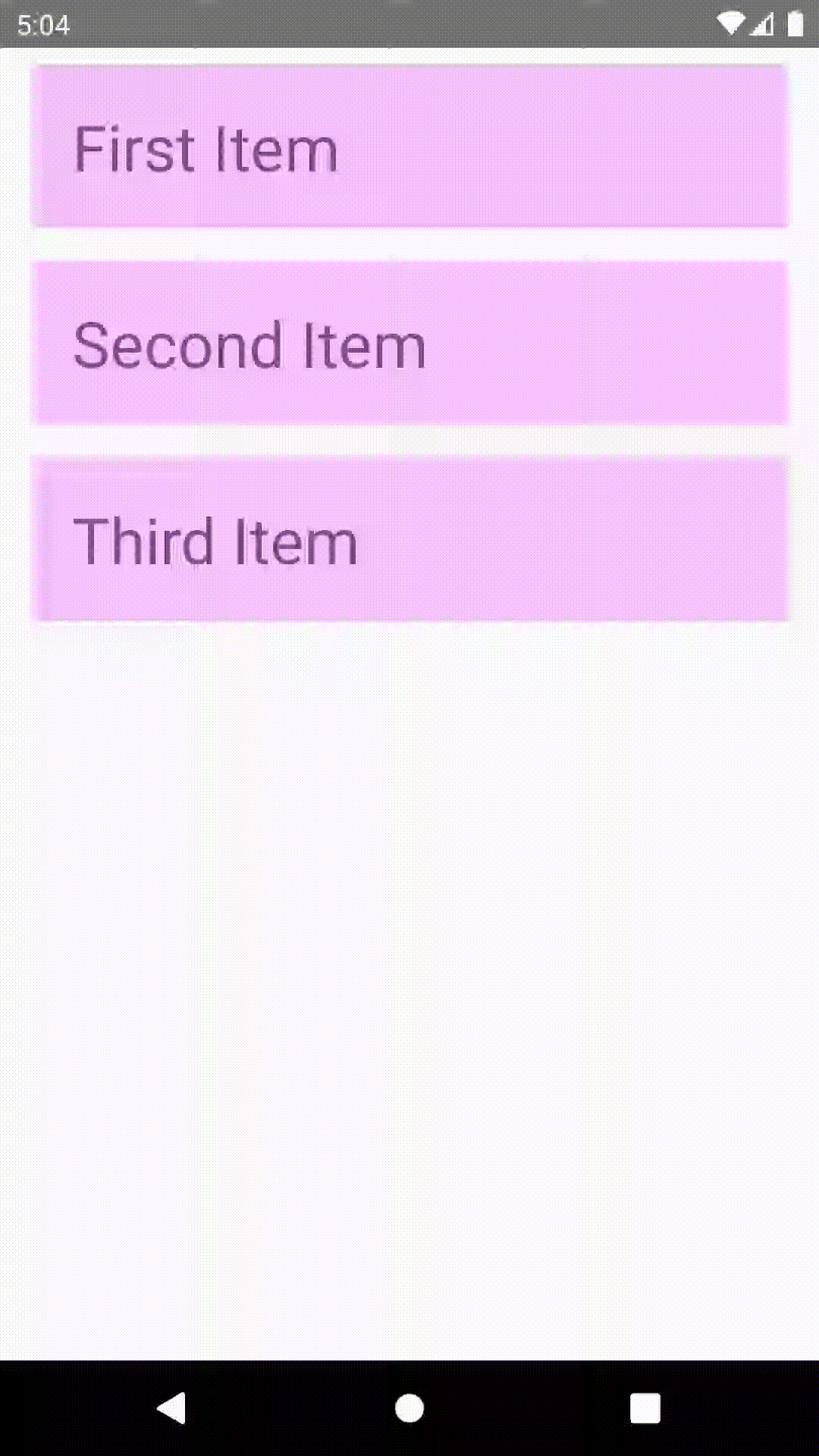
I hope this react native tutorial to drag and reorder FlatList items is helpful for you.
In the above example, the following versions are used.
“react-native”: “0.67.4”,
“react-native-draggable-flatlist”: “^3.0.7”,
“react-native-gesture-handler”: “^2.3.2”,
“react-native-reanimated”: “^2.5.0”