How to Disable TextInput in React Native
In mobile apps, it’s common to require form controls that can be enabled or disabled based on certain conditions. One such control is the TextInput
in React Native.
In this blog post, we will go through the ways you can disable TextInput
and scenarios where this might be useful.
Prerequisites
- A basic understanding of React Native
- A React Native development environment set up
The Basics: Disabling TextInput
Disabling TextInput
in React Native is pretty straightforward. You just need to set the editable
prop to false
.
import React from 'react';
import {TextInput, View, StyleSheet} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<TextInput
style={styles.input}
editable={false}
placeholder="This input is disabled"
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
input: {
height: 40,
borderColor: 'gray',
borderWidth: 1,
backgroundColor: '#f2f2f2',
},
});
export default App;
We import the required modules. The editable
prop is set to false
to disable the input field. The backgroundColor
is set to #f2f2f2
to make the input field appear grayed out.
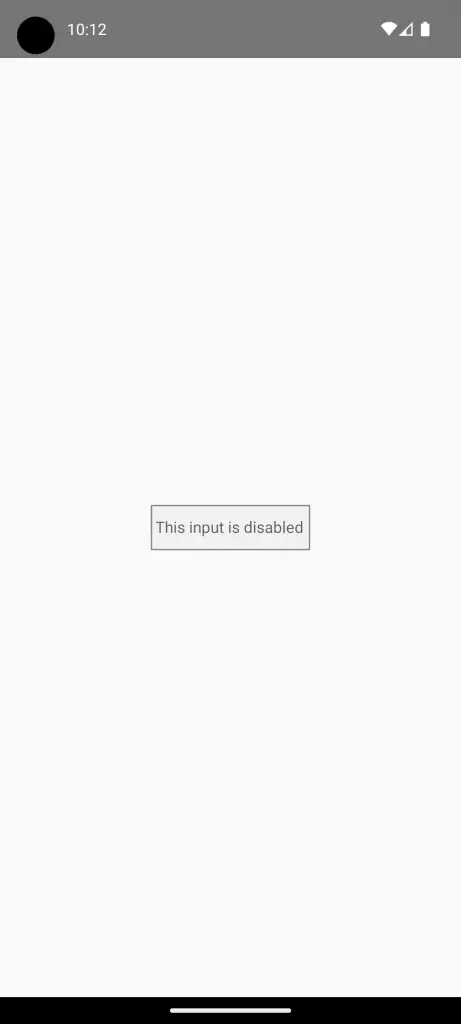
Conditionally Disable TextInput
There are scenarios where you’d want to disable the TextInput
based on certain conditions.
const App = () => {
const [isDisabled, setDisabled] = React.useState(false);
return (
<View style={styles.container}>
<TextInput
style={styles.input}
editable={!isDisabled}
placeholder="Conditionally disabled"
/>
</View>
);
};
We use useState
to manage the disabled state of TextInput
. The editable
prop is dynamically set based on the isDisabled
state variable.
Use Cases
- Form Submission: Disable input fields while submitting a form to prevent changes.
- User Permissions: Disable fields based on the user’s role or permissions.
- Data Loading: Disable input fields while waiting for data to load.
Disabling TextInput
in React Native is a straightforward process. You just need to use the editable prop of the TextInput component.