How to Check Internet Connectivity in React Native (Android and iOS)
Most of the mobile apps in the market use the internet to provide services. Hence listening to internet connectivity changes is very important to make the user aware of the situation.
In react native, NetInfo API was used to test whether the Internet is connected or not. Now, NetInfo is not part of react native core and hence you should use react native NetInfo library.
You can install the library using any of the following commands.
yarn add @react-native-community/netinfo
or
npm install --save @react-native-community/netinfo
For iOS devices, run the following command too.
npx pod-install
If you are using react native expo then install the library using the instructions given here.
In order to check connectivity the easy way is to import the useNetInfo hook and use it as given below.
import {useNetInfo} from '@react-native-community/netinfo';
const netInfo = useNetInfo();
const checkConnection = () => {
if (netInfo.isConnected && netInfo.isInternetReachable) {
Alert.alert('You are online!');
} else {
Alert.alert('You are offline!');
}
};
The isConnected property checks for an active network connection whereas the isInternetReachable property checks whether an active internet connection available in the network.
Following is the complete code where the connectivity is checked when the Pressable component is pressed.
import React from 'react';
import {StyleSheet, Alert, Text, View, Pressable} from 'react-native';
import {useNetInfo} from '@react-native-community/netinfo';
const App = () => {
const netInfo = useNetInfo();
const checkConnection = () => {
if (netInfo.isConnected && netInfo.isInternetReachable) {
Alert.alert('You are online!');
} else {
Alert.alert('You are offline!');
}
};
return (
<View style={styles.container}>
<Pressable
onPress={() => {
checkConnection();
}}>
<Text>Check Connectivity</Text>
</Pressable>
<Text>Type: {netInfo.type}</Text>
<Text>Is Connected? {netInfo.isConnected?.toString()}</Text>
<Text>
Is Internet Available? {netInfo.isInternetReachable?.toString()}
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Following is the output.
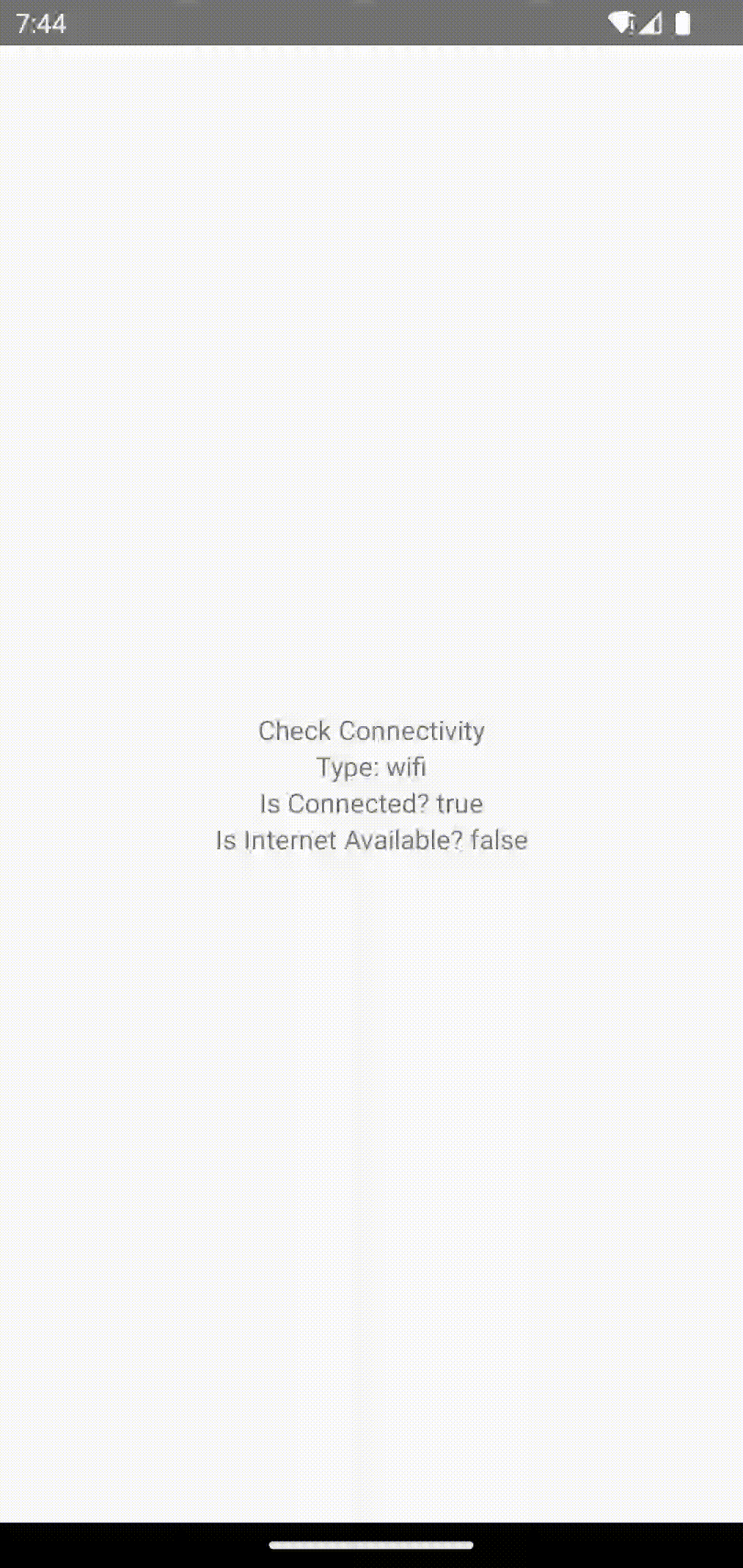
That’s how you check internet connectivity in React Native.
I have tested, but the problem this not check your internet connection, only the wifi. If you are connected by wifi, but not have internet in your router, the NETInfo will return true.
That’s why a good idea would be to “ping” some endpoint in a API rest, maybe create a api.host.com/healthcheck to access and get the return parameters.