How to Add Border to Row in Flutter
In Flutter, adding a border to a Row
widget can enhance the visual structure of your app’s interface. Whether you’re highlighting a section or just creating a neat, organized look, borders can be quite useful.
However, since the Row
widget itself doesn’t have a property for borders, we need to use a combination of other widgets to achieve this effect. Here’s how you can add a border to a Row
in Flutter.
Wrap Row in a Container
The easiest way to add a border to a Row
is to wrap it in a Container
widget, which does come with a decoration property.
Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.blue),
),
child: Row(
children: [
Text('This is a sample row with a colorful border!')
],
),
)
The BoxDecoration
allows us to add a border with the desired color and width.
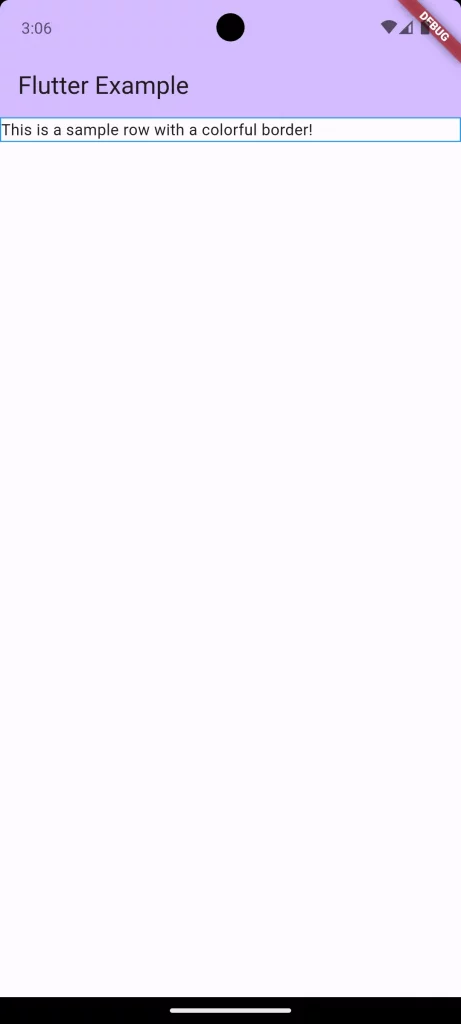
Customize the Border
You can further customize the border by changing its color, width, and style. For example, if you want a dashed border or a border with rounded corners, you’d adjust the BoxDecoration
like so:
Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.blue, width: 2, style: BorderStyle.solid),
borderRadius: BorderRadius.circular(12),
),
child: const Row(
children: [
Text('This is a sample row with a colorful border!')
],
),
)
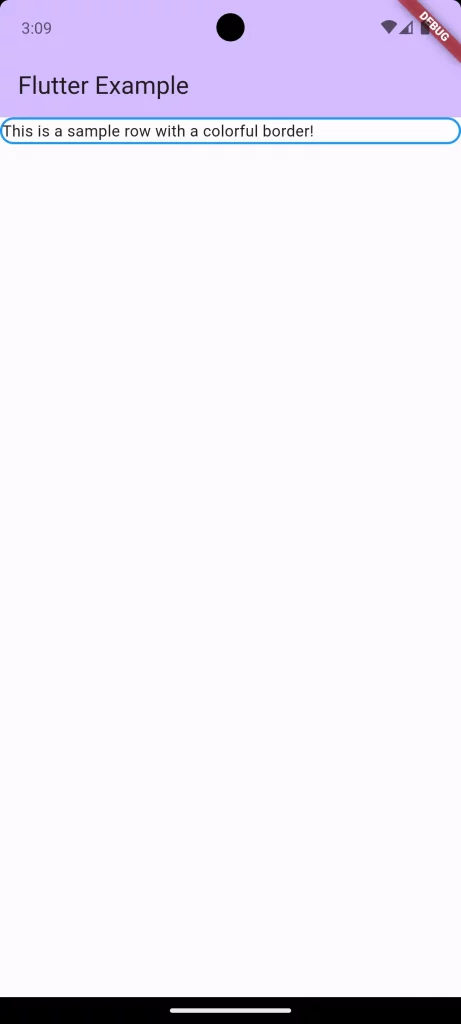
Apply Padding Inside the Border
Often, you’ll want some spacing between the border and the Row
‘s children. You can achieve this by setting the padding property of the Container
.
Container(
padding: const EdgeInsets.all(8.0), // Add padding inside the container
decoration: BoxDecoration(
border: Border.all(color: Colors.blue),
),
child: const Row(
children: [
Text('This is a sample row with a colorful border!')
],
),
)
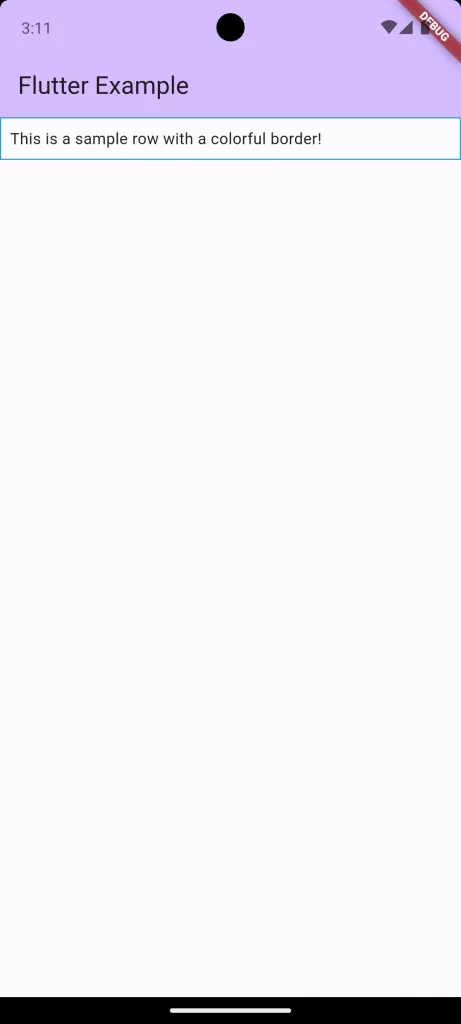
Deal with Margins Outside the Border
If you need some space outside the border, use the margin property of the Container
. This will add space between the border and any adjacent widgets.
Container(
margin: EdgeInsets.all(8.0), // Add margin outside the container
// ...other properties...
)
Adding a border to a Row
in Flutter may involve a few additional steps, but it’s a straightforward process. By wrapping your Row
in a Container
and utilizing the BoxDecoration
class, you can add and customize borders as needed.
Remember to consider padding, margins, and overflow, especially when dealing with multiple screen sizes. With these tips, you can enhance the design of your Flutter application, using borders to create clean, attractive layouts that improve user experience.