How to Show URL Previews in React Native
If you have a mobile app that has link sharing capabilities then showing URLs or link previews is very helpful for the users. We see this feature in popular apps such as WhatsApp.
To show URL previews in react native apps, I prefer react native link preview library. It’s a simple library and you can install it in your project with the following command.
yarn add @flyerhq/react-native-link-preview
It’s pretty simple to generate link previews with the RNUrlPreview component.
import { LinkPreview } from '@flyerhq/react-native-link-preview'
// ...
return (
<LinkPreview text='This link https://github.com/flyerhq can be extracted from the text' />
)
You can see all the available props here.
Here’s the complete example to show URL previews in react native.
import React from 'react';
import {View, StyleSheet} from 'react-native';
import {LinkPreview} from '@flyerhq/react-native-link-preview';
const App = () => {
return (
<View style={styles.container}>
<LinkPreview
containerStyle={styles.previewContainer}
enableAnimation
text="https://pinterest.com"
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
previewContainer: {
width: '80%',
backgroundColor: '#f7f7f8',
borderRadius: 20,
overflow: 'hidden',
},
});
export default App;
Here’s the output of the react native example.
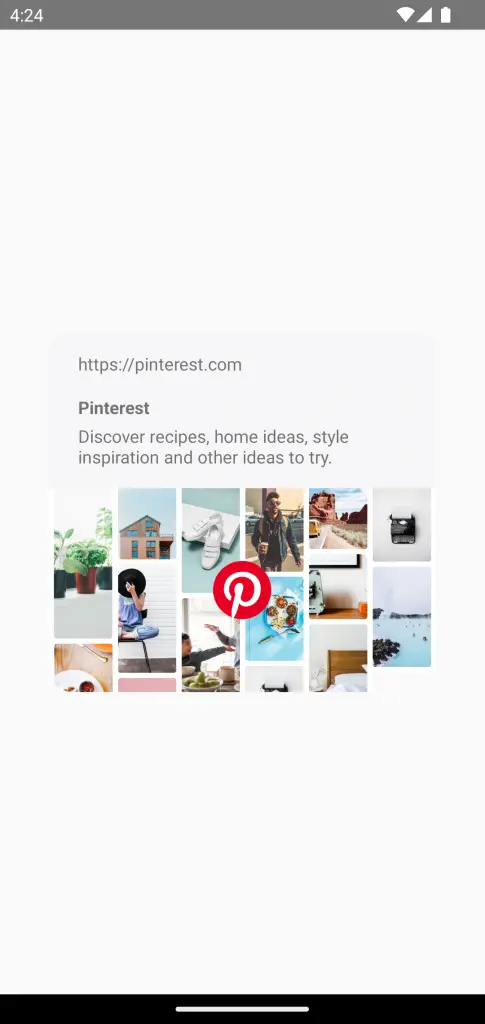
Thank you for reading!!