How to Check Android API Version in React Native
If you are developing an Android app using React Native then you may need to know the Android version of your Android app for various version-specific purposes.
For example, if you need to prompt running permissions in Android API versions above Android Lollipop. For this, you should know the Android API level of the user’s device.
You can do this by simply using the Platform API of react native. The Platform.Version gives the API version number.
import React from 'react';
import {StyleSheet, Text, View, Platform} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text>API Version {Platform.Version?.toString()}</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Following is the output.
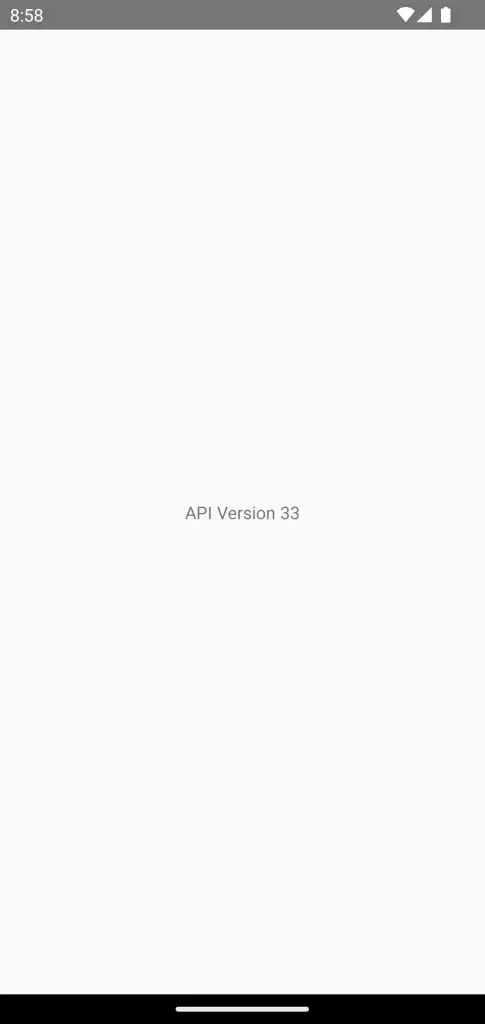
That’s how you check Android API version in react native.
Can we init an project with a specific Android Api level ?