How to Highlight Text in React Native
Highlighting text can be a crucial feature in apps for improving readability or drawing the user’s attention to specific parts of content. Previously, we looked at how to highlight specific words of text. This time, we’ll explore how to highlight all the words in a given text string using React Native.
Prerequisites
- Basic knowledge of JavaScript and React Native
- An existing React Native project to work on
The Code Example and Explanation
Below is the example code:
import React from 'react';
import { StyleSheet, View, Text } from 'react-native';
const highlight = string =>
string.split(' ').map((word, i) => (
<Text key={i}>
<Text style={styles.highlighted}>{word} </Text>
</Text>
));
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
{highlight("Here is some text that should be entirely highlighted.")}
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 24,
justifyContent: 'center',
},
text: {
fontSize: 20,
},
highlighted: {
backgroundColor: 'yellow',
},
});
export default App;
The highlight
Function
The function highlight
is our key player here. It takes a string and then:
- Splits it into an array of words using JavaScript’s
split
method. - Maps over the array, wrapping each word with a
Text
component that has ahighlighted
style.
Essentially, all the words in the text will be highlighted using this function.
The App
Component
Our main component, App
, calls the highlight
function to process the text. This results in each word of the sentence “Here is some text that should be entirely highlighted.” being highlighted.
Styling
For the visual aspect of highlighting, we have defined a highlighted
style within a StyleSheet
object. This style sets the background color of each word to yellow.
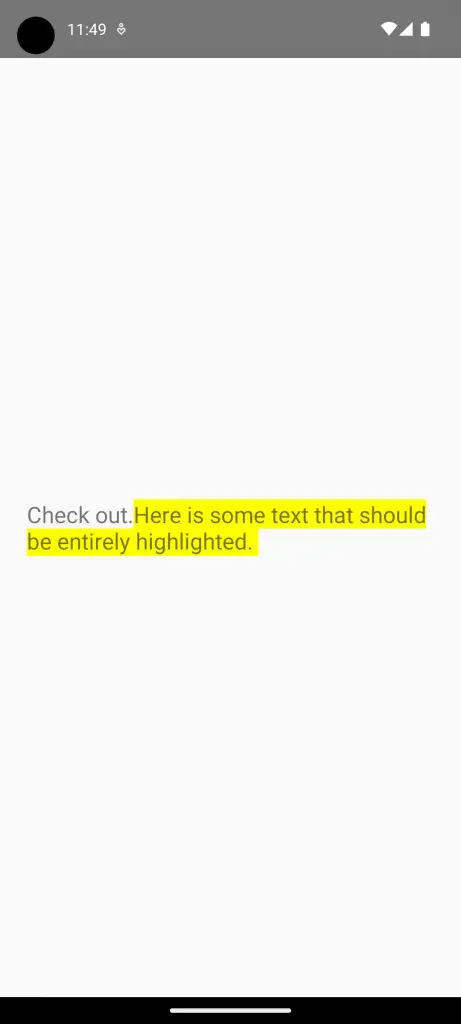
With a straightforward function, you can highlight all words within a text string in a React Native application. The example above shows how to apply this concept effectively and you can easily adapt it for your specific needs.