How to Display Gif Images in React Native Android App
You can easily display gif images in ios but things are not the same in Android. In a normal straightforward case, the following snippet will work on ios but not on Android devices.
<Image source={{uri: 'http://www.yourimageurl.com/image.gif'}} />
Now, assume that I want to show the following gif from the giphy website. It’s a gif image in the webp format.
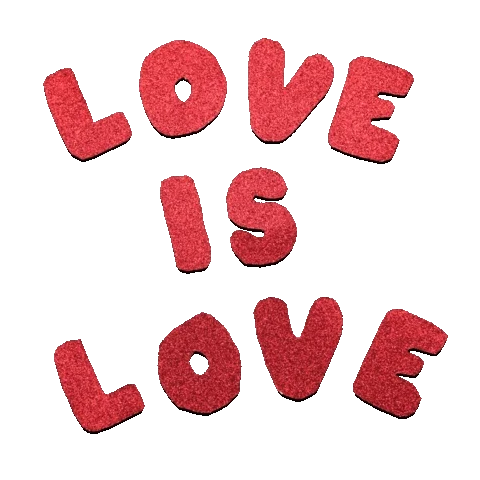
In order to get gif support on Android devices, you need to use the dependencies of Facebook’s Fresco library. Open YourProject>Android>App>build.gradle file and add the following lines to dependencies {} tag.
// For animated GIF support
implementation 'com.facebook.fresco:animated-gif:2.5.0'
// For WebP support, including animated WebP
implementation 'com.facebook.fresco:animated-webp:2.5.0'
As given in the comments, the dependencies are for gif support as well as for the animated webp format. If you want to know information about dependencies go here.
After adding the dependencies, the following code will work on Android devices. Don’t forget to run the project after adding dependencies using npx react-native run-android command.
import {StyleSheet, Image, View} from 'react-native';
import React from 'react';
const App = () => {
return (
<View style={styles.container}>
<Image
style={styles.image}
source={{
uri: 'https://media2.giphy.com/media/MaaaYoyYTMjuIct0wD/giphy.webp',
}}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
image: {
height: 250,
width: 250,
},
});
export default App;
The output of react native example for gif support in Android os will be as given below:

That’s how you add gif support to react native android apps!
Making GIF is very difficult. But After reading your article i can solve my problem.This code is very helpful to us. I think I got all the information I was looking for. Thanks for this wonderful piece of article.
It was very hard for me to create various kind of GIF’s. After reading your article I found all the tips very much useful. Thanks for posting blogs like this.
great tutorial also if your want to use some thrid-party gif like Giphy I recommend check out my post
https://www.instamobile.io/mobile-development/giphy-react-native/
Thank
get gif support on Android devices, you need to use the dependencies of Facebook’s Fresco library. Keep posting more articles on GIF.