How to Disable Picker Component in React Native
The Picker component is a controlled component, which means that the user’s selection is controlled by the React Native component. Sometimes, you may need to disable the picker component to avoid any further actions related to the same.
In order to disable the Picker component in React Native you just need to use its enabled prop. Set enabled as false to make the picker disabled. This will disable the Picker and prevent the user from making any selections.
Like the example given below.
<Picker
selectedValue={language}
style={{height: 50, width: 100}}
enabled={false}
prompt="Choose Language"
onValueChange={(itemValue, itemIndex) => setlanguage(itemValue)}>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
You will get a disabled Picker component as output.
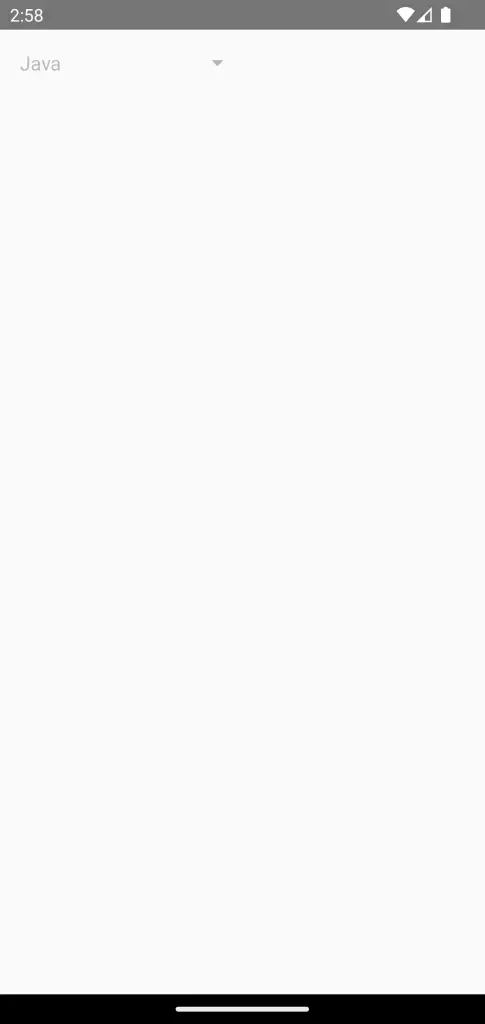
Following is the complete code.
import React, {useState} from 'react';
import {View} from 'react-native';
import {Picker} from '@react-native-picker/picker';
const App = () => {
const [language, setlanguage] = useState('java');
return (
<View>
<Picker
selectedValue={language}
style={{height: 50, width: 200}}
enabled={false}
prompt="Choose Language"
onValueChange={(itemValue, itemIndex) => setlanguage(itemValue)}>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
</View>
);
};
export default App;
That’s how you disable Picker in react native.
enabled={false} for the picker is not working