How to Create Dotted Underlined Text in Flutter
Even though very rare sometimes you may want to underline a text with a dotted line. Let’s check how to do that in Flutter.
You may also want to check out my other blog posts – how to underline text in Flutter and how to double underline text in Flutter.
Using the TextStyle widget you can make TextDecorationStyle dotted to create a dotted underline in Flutter.
See the code snippet given below.
const Text(
'Dotted Underline',
style: TextStyle(
color: Colors.black
decoration: TextDecoration.underline,
decorationStyle: TextDecorationStyle.dotted,
),
)
If you only want to make some specific text to be underlined then better choose the RichText widget over the normal Text Widget.
See the Flutter example given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Dotted Underline Text',
),
),
body: Center(
child: RichText(
text: const TextSpan(
text: 'Here is ',
style: TextStyle(fontSize: 40, color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'Dotted',
style: TextStyle(
decoration: TextDecoration.underline,
decorationStyle: TextDecorationStyle.dotted,
)),
TextSpan(text: ' underlined text!'),
],
),
)));
}
}
Following is the output of the above example.
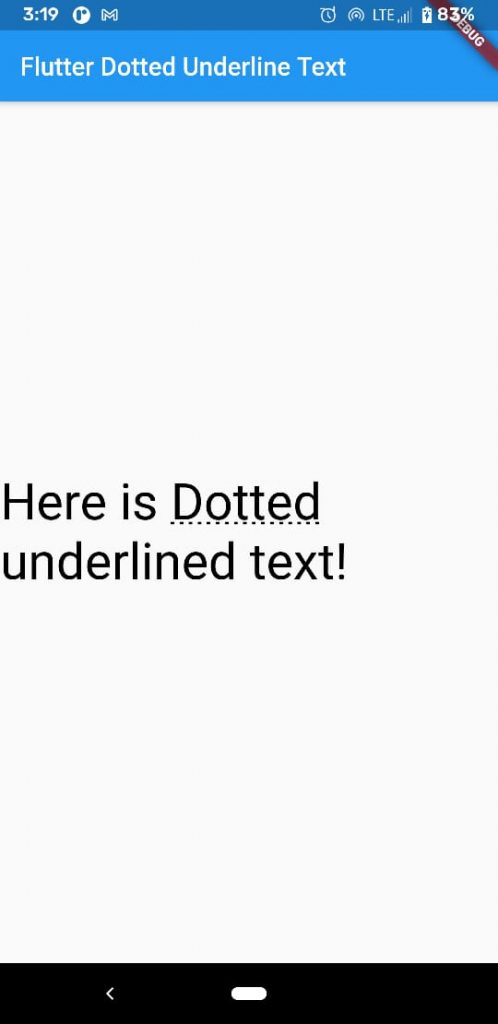
I hope this Flutter tutorial will be helpful for you.