How to Create a Circle Shape in Flutter
Sometimes, the user interface of a mobile app can be complex. We may need to add custom shapes for design purposes. In this Flutter example, Let’s see how to create a round shape in flutter easily.
You can create a circle in flutter easily using Container, BoxDecoration, and BoxShape widgets as given below.
Container(
width: 300.0,
height: 300.0,
decoration: const BoxDecoration(
color: Colors.blue,
shape: BoxShape.circle,
),)
Following is the complete Flutter circle shape example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Circle Example',
),
),
body: Center(
child: Container(
width: 300.0,
height: 300.0,
decoration: const BoxDecoration(
color: Colors.blue,
shape: BoxShape.circle,
),
),
),
);
}
}
The output is given below.
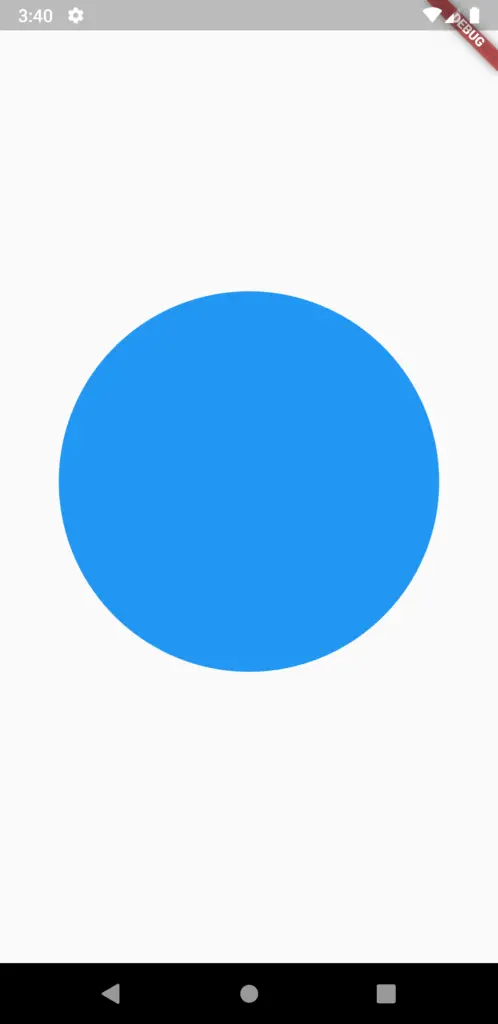
I hope this simple flutter tutorial will be helpful for you.