How to use .env File in React Native
Environment variables are global variables. The files with the .env extension are suitable for saving variables such as domains, API URLs, endpoints, etc. They help to decouple configuration details from our whole project.
How to setup .env file in react native
In react native, just adding a .env file is not enough. You have to load environment variables using the import statement. The react native dotenv library helps to configure and import the environment variables in react native.
First of all, install the library using the following command.
npm install -D react-native-dotenv
Create a new file named .babelrc in the project folder and add the following snippet to it.
{
"plugins": [
["module:react-native-dotenv"]
]
}
Create another file named .env in your project folder. Then add two global variables in it as follows.
API_KEY=test
LOCAL_CONFIG=environmentVariable
You can access these variables from other files by importing them.
import {API_KEY, LOCAL_CONFIG} from "@env";
For example, you can show the environment variables as text (only for demonstration).
import React from 'react';
import {Text, View} from 'react-native';
import {API_KEY, LOCAL_CONFIG} from '@env';
const App = () => {
return (
<View>
<Text>{API_KEY}</Text>
<Text>{LOCAL_CONFIG}</Text>
</View>
);
};
export default App;
Then you will get the following output.
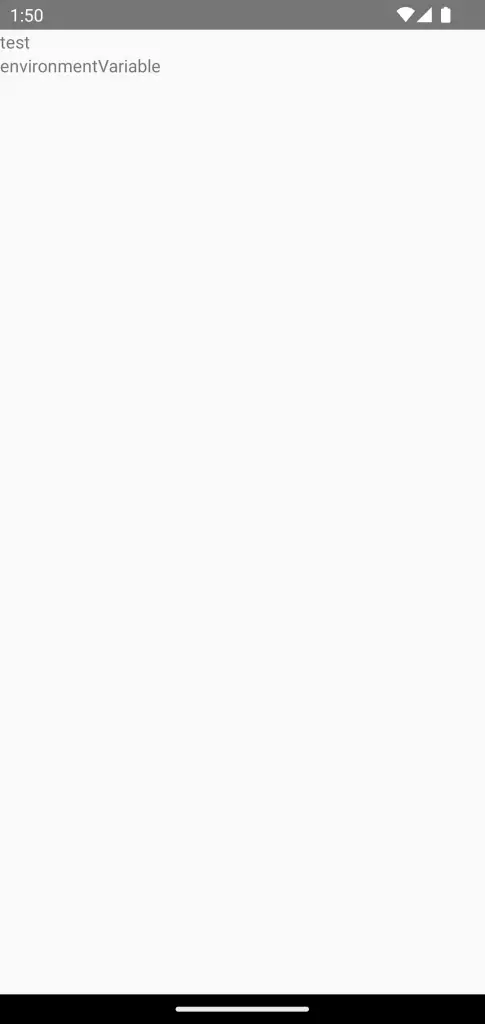
This is just a basic setup of the .env file in react native. You can tweak advanced configurations by following the instructions given here.
That’s how you set up environment variables in react native easily.