How to Remove Debug Banner in Flutter
By default, when you run your projects in debug mode Flutter shows a debug banner on the top right of the app bar. In this tutorial let’s learn how to hide this debug banner in Flutter.
Following is basic Flutter example with a Text placed at the center of the screen.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Hide Debug banner in Flutter',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
const FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Remove Debug Banner in Flutter')),
body: Center(child: Text('Flutter Example')));
}
}
You can see the debug label at the top of the screen.
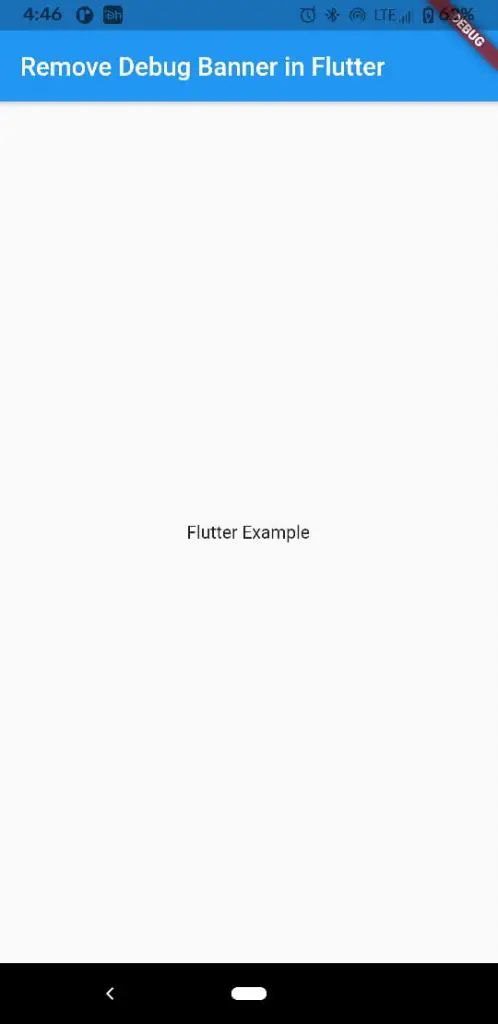
Now, let’s set debugShowCheckedModeBanner property as false on MaterialApp class. See the code given below.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Hide Debug banner in Flutter',
debugShowCheckedModeBanner: false,
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
const FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Remove Debug Banner in Flutter')),
body: Center(child: Text('Flutter Example')));
}
}
See the output now.
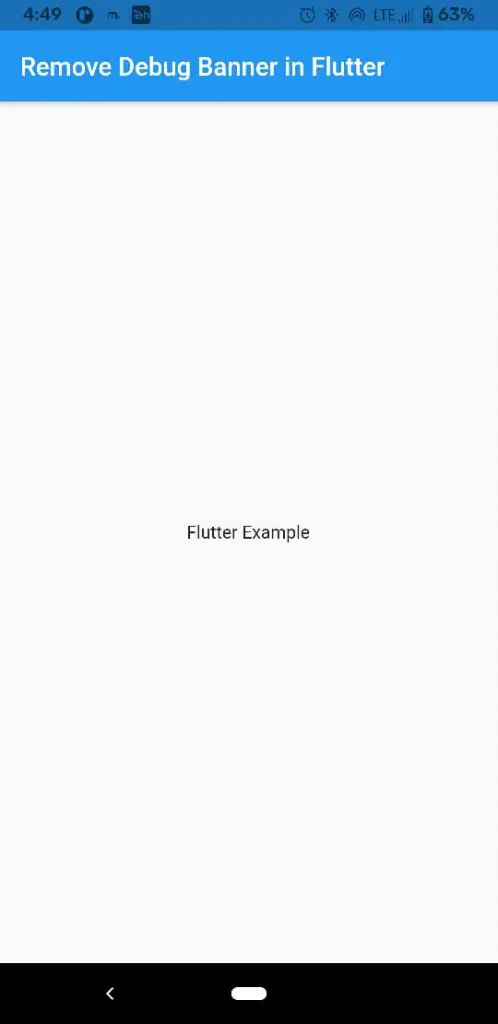
That’s how you remove debug label in Flutter.