How to Make Inline Text Bold in React Native
Text styling is a crucial part of any mobile application’s UI. One common requirement is making certain words or phrases in a text string bold, while keeping others in regular style. In this tutorial, we’ll focus on how to achieve inline text styling, specifically making text bold, in a React Native application.
Prerequisites
- A React Native development environment set up
- Basic understanding of JavaScript and React Native
Simple Inline Text Styling
The simplest way to make inline text bold in React Native is by using nested Text
components.
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text>
This is <Text style={styles.boldText}>bold</Text> text.
</Text>
</View>
);
};
The Text
component allows nesting. We wrap the word “bold” in another Text
component and apply styling.
Styling
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
boldText: {
fontWeight: 'bold',
},
});
Complete Code
Following is the complete code.
import React from 'react';
import {Text, View, StyleSheet} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text>
This is <Text style={styles.boldText}>bold</Text> text.
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
boldText: {
fontWeight: 'bold',
},
});
export default App;
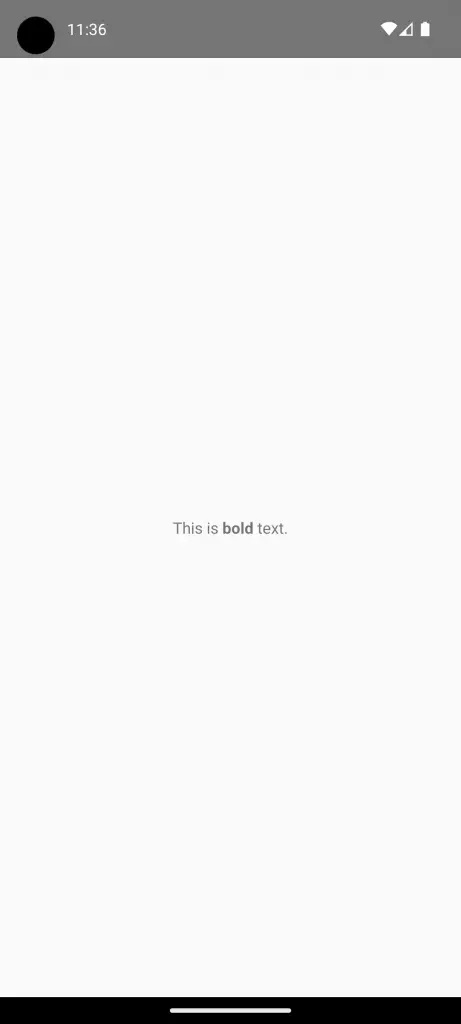
React Native provides multiple ways to make inline text bold. You can choose to use nested Text
components, arrays, or even functions for more dynamic needs. Each method has its own use-cases and advantages, making inline text styling in React Native both flexible and powerful.