How to Align Button to Bottom of the Screen in React Native
While designing the UI of your app sometimes you may want to place your button at the bottom of the screen. In such cases, you can use the power of the flexbox so that the button would be aligned to the bottom of the screen irrespective of screen size.
I have written a sample code below which shows how a button should be aligned to the bottom. I think the things are self-explanatory in the code.
import React from 'react';
import {View, Text, TouchableOpacity, StyleSheet} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
Hey, that's how you show button at the bottom of the screen!
</Text>
<View style={styles.bottom}>
<TouchableOpacity style={styles.button}>
<Text style={styles.buttonText}>Bottom Button</Text>
</TouchableOpacity>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
bottom: {
flex: 1,
justifyContent: 'flex-end',
},
button: {
width: '100%',
height: 50,
backgroundColor: 'red',
alignItems: 'center',
justifyContent: 'center',
},
buttonText: {
fontSize: 16,
color: 'white',
},
text: {
margin: 20,
color: 'black',
},
});
export default App;
Following is the output.
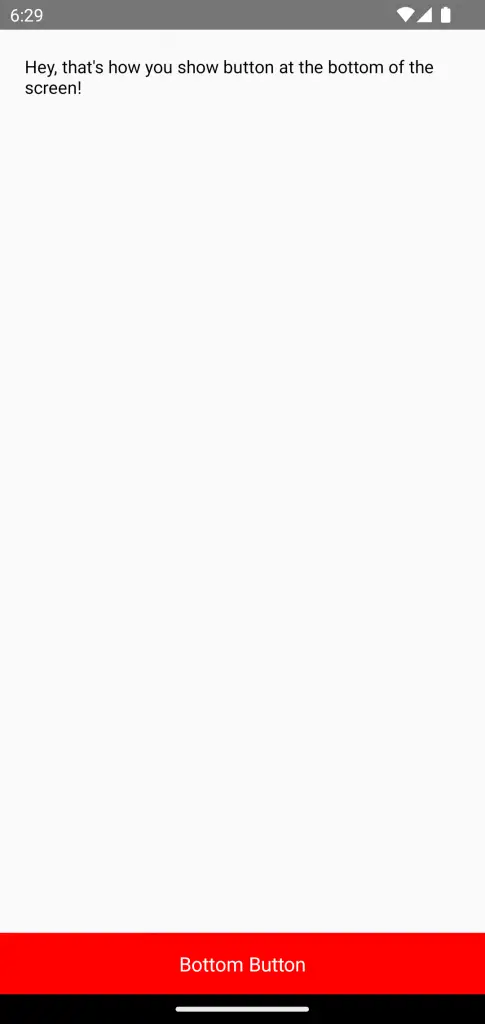
That’s how you display a button at the bottom of the screen in react native.