How to make Text Clickable in React Native
Sometimes, you may want to make a text clickable in order to perform an action when it is clicked. This can be useful for creating interactive and user-friendly apps. In this blog post, let’s learn how to make the Text clickable in react native.
To make text clickable, you can wrap it in either a Pressable component or a TouchableOpacity component. Then you just need to assign onPress to define what happens after the click.
See the code snippet given below.
<Pressable onPress={() => Alert.alert('The text is clicked!')}>
<Text>This is clickable text</Text>
</Pressable>
Following is the output.
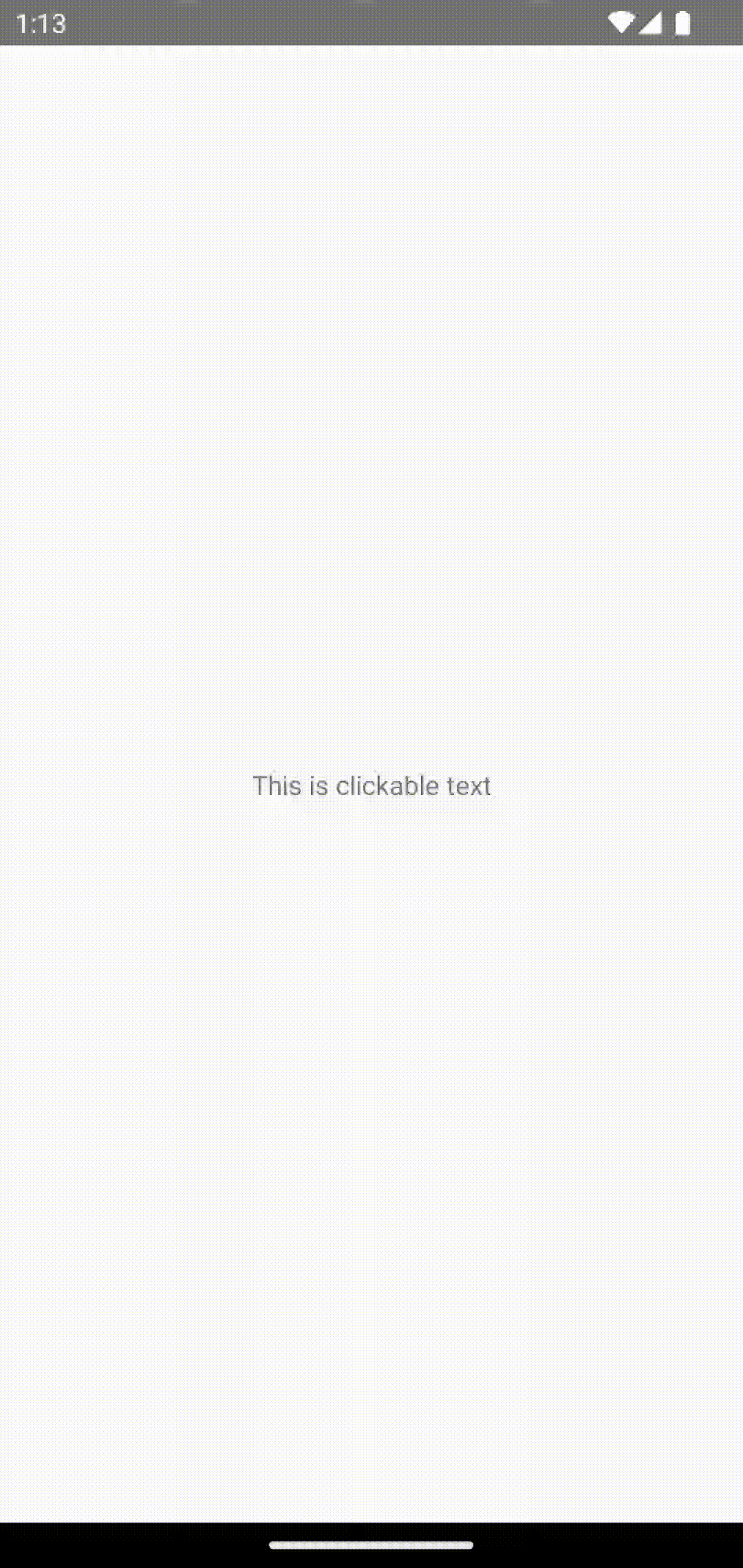
Following is the complete code.
import React from 'react';
import {View, Text, Alert, Pressable, StyleSheet} from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Pressable onPress={() => Alert.alert('The text is clicked!')}>
<Text>This is clickable text</Text>
</Pressable>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
That’s how you make text clickable in react native.